i2ctransfer -f -y 8 w40x68 0x32 0x2 0x04 0xle r5 向设备地址0x68中写入4个数据,随后读取5个数据 对应的代码
时间: 2024-02-20 22:56:43 浏览: 18
这段命令对应的是使用 i2c 总线向从设备地址为 0x68 的设备写入 4 个数据,然后读取 5 个数据。
下面是使用 C 语言编写的 i2c 读写数据的代码示例:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <linux/i2c-dev.h>
#include <sys/ioctl.h>
int main(void) {
int fd;
char *filename = "/dev/i2c-1"; // i2c 总线设备文件路径
int address = 0x68; // 从设备地址
char tx_buf[5] = {0x32, 0x02, 0x04, 0x1e}; // 写入数据缓冲区
char rx_buf[5]; // 读取数据缓冲区
// 打开 i2c 总线设备文件
if ((fd = open(filename, O_RDWR)) < 0) {
perror("Failed to open i2c bus");
return 1;
}
// 设置从设备地址
if (ioctl(fd, I2C_SLAVE, address) < 0) {
perror("Failed to set i2c address");
return 1;
}
// 写入数据
if (write(fd, tx_buf, 4) != 4) {
perror("Failed to write data");
return 1;
}
// 读取数据
if (read(fd, rx_buf, 5) != 5) {
perror("Failed to read data");
return 1;
}
// 输出读取的数据
printf("Read data: ");
for (int i = 0; i < 5; i++) {
printf("%02x ", rx_buf[i]);
}
printf("\n");
// 关闭 i2c 总线设备文件
close(fd);
return 0;
}
```
其中,需要注意的是:
- 需要先使用 `open` 函数打开 i2c 总线设备文件,并设置总线地址;
- 使用 `ioctl` 函数设置从设备地址;
- 使用 `write` 函数向设备写入数据;
- 使用 `read` 函数从设备读取数据;
- 读取的数据长度需要与命令中的 `r5` 对应。
相关推荐
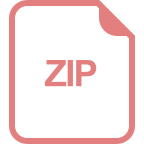
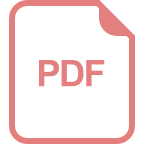





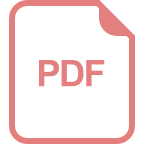
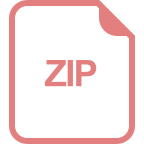
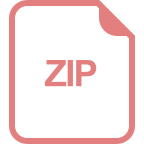
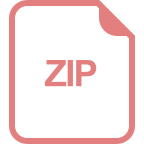
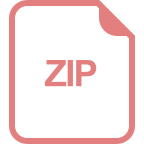
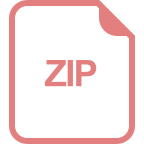
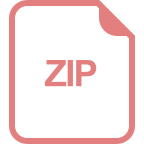
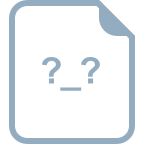
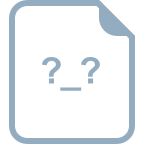