var name=<%request.getServletContext().getAttribute("name");%>这句话对吗
时间: 2024-03-21 12:42:07 浏览: 19
不完全正确。
这里的代码 `<%request.getServletContext().getAttribute("name");%>` 是 JSP 页面中的脚本表达式,它可以在页面中嵌入 Java 代码,并将其执行结果输出到 HTML 页面中。在这个表达式中,我们通过 `request.getServletContext().getAttribute("name")` 方法获取了 ServletContext 中名为 `name` 的属性值,但是我们没有将其赋值给前端的 `var` 对象。
如果我们想要将 ServletContext 中的属性值赋值给前端的 `var` 对象,可以将这个表达式的值赋给 `var` 对象,即:
```html
<script>
var name = "<%= request.getServletContext().getAttribute("name") %>";
</script>
```
在这个代码中,我们将 `request.getServletContext().getAttribute("name")` 的返回值赋值给前端的 `var` 对象 `name`。注意,在这里我们需要使用 `"<%= %>"` 这种方式来将表达式的执行结果输出到 HTML 页面中。同时,由于属性值可能是字符串类型,因此我们需要使用双引号将其包裹起来。
相关问题
这句话有错误吗<img src=<%=request.getServletContext().getAttribute("lujing")%>>
是的,这句话有错误。它似乎是一个Java Web应用程序中的JSP代码,但是在这里它没有上下文,所以无法正常工作。在正确的环境中,它应该被包含在JSP标记中,并且<%=request.getServletContext().getAttribute("lujing")%>应该被正确的替换为一个合适的值。
这个jsp代码路径正确,为什么获取不到图片<img src="<%=request.getServletContext().getAttribute("lujing")%>">
可能是因为获取到的路径不正确导致无法获取图片。
您可以尝试使用以下代码来获取当前Web应用程序的根目录,然后将图片路径与之拼接:
```jsp
<%
String contextPath = request.getContextPath();
String imagePath = (String) request.getServletContext().getAttribute("lujing");
String imgUrl = contextPath + imagePath;
%>
<img src="<%=imgUrl%>">
```
这样可以确保生成正确的图片路径。如果仍然无法显示图片,可能是因为图片文件不存在或者没有足够的权限访问该文件。
相关推荐
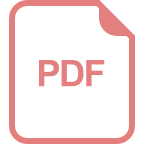
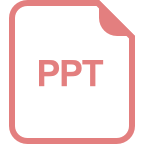




<%-- 作者:GGG166 --%> <%@page contentType="text/html;charset=gb2312" %> <%@page import="java.util.*"%> <html> <body> <%! Vector v=new Vector();//动态数组 int i=0; ServletContext application; synchronized void leaveWord(String s){//方法声明,用于在添加评论 application=getServletContext(); i++; v.add("No"+i+","+s); application.setAttribute("Mess",v); } %> <% request.setCharacterEncoding("gb2312");//乱码处理 String name=request.getParameter("name");//接收姓名 String title=request.getParameter("title");//接收标题 String message=request.getParameter("message");//接收评论 if(name==null){ name="guest"+(int)(Math.random()*10000); } if(title==null){ title="无标题"; } if(message==null){ message="无信息"; } String s=name+"#"+title+"#"+message; leaveWord(s); out.print("你的评论已提交!"); %> 返回留言页面 </body> </html>








