cpp-httplib基本使用
时间: 2023-03-27 13:02:24 浏览: 807
cpp-httplib是一个C++编写的HTTP客户端/服务器库,可以用于快速开发HTTP客户端和服务器。它提供了简单易用的API,支持GET、POST、PUT、DELETE等HTTP方法,同时也支持SSL加密连接。使用cpp-httplib可以轻松地实现HTTP通信功能。
相关问题
CPP-httplib
### 关于C++ httplib库的使用和文档
httplib 是一个轻量级的HTTP服务器和客户端C++库,旨在简化HTTP通信开发工作。此库支持GET、POST等多种请求方法以及文件上传等功能。
#### 安装与配置
为了使用httplib,在项目中引入头文件即可开始:
```cpp
#include "httplib.h"
```
通常情况下不需要额外链接其他动态或静态库,因为httplib依赖于标准C++库实现网络功能[^1]。
#### 创建简单的HTTP服务器实例
下面是一个创建基本HTTP服务器的例子,该服务器监听8080端口并响应根路径下的任何请求:
```cpp
#include <iostream>
#include "httplib.h"
int main() {
httplib::Server svr;
svr.Get("/", [](const httplib::Request&, httplib::Response& res) {
res.set_content("Hello World", "text/plain");
});
std::cout << "Starting server on :8080...\n";
svr.listen("localhost", 8080);
}
```
这段代码定义了一个处理程序来回应来自`/`路径的所有GET请求,并返回字符串"Hello World"[^1]。
#### 发送HTTP GET 请求作为客户端
如果想要通过C++发送HTTP GET请求,则可以这样做:
```cpp
#include <iostream>
#include "httplib.h"
int main() {
auto res = httplib::get("http://example.com");
if (res && res->status == 200) {
std::cout << res->body;
} else {
std::cerr << "Error during request\n";
}
}
```
这里展示了如何利用httplib发起针对指定URL的GET请求,并打印出收到的内容体部分。
cpp实现从cpp-httplib server获取本地文件
在C++中,你可以使用cpp-httplib库来作为HTTP服务器,然后通过发送GET请求从这个服务器下载本地文件。以下是基本步骤:
1. 首先,确保你已经安装了cpp-httplib库。如果没有,可以从GitHub上克隆并添加到项目中:https://github.com/yhirose/cpp-httplib
2. 引入必要的头文件:
```cpp
#include <cpp-httplib.h>
```
3. 定义一个函数来处理HTTP GET请求,该函数将检查请求的目标是否是你想要提供下载的本地文件路径:
```cpp
void download_file(const std::string& filename) {
htp::server my_server(8080); // 设置服务器监听的端口
auto file_path = "path/to/your/local/file.txt"; // 替换为你实际的文件路径
if (httplib::OK == my_server.get("/download", [&](const htp::request& r) -> htp::response {
if (r.uri.path() == "/download" && r.method == htp::Method::GET) {
std::ifstream in(file_path);
if (!in) {
return htp::response(404, "File not found");
}
htp::header headers;
headers["Content-Type"] = "text/plain";
headers["Content-Disposition"] = "attachment; filename=" + filename;
return htp::response(200, "OK", in, headers);
}
return htp::response(httplib::Status::NotImplemented, "Not implemented");
})) {
std::cout << "Server running on port 8080." << std::endl;
} else {
std::cerr << "Failed to start the server: " << my_server.status_message() << std::endl;
}
}
```
4. 启动服务器:
```cpp
int main() {
download_file("file_to_download.txt"); // 调用下载文件函数,并指定文件名
try {
my_server.run();
} catch (std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
阅读全文
相关推荐

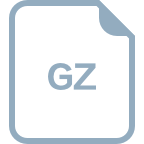

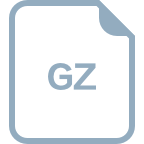










