学生管理系统 学生Student(属性封装){ id:Long唯一 学号:String,唯一 姓名:String 电话:String,唯一 年龄:Integer 性别:Integer、Boolean 爱好:String[] 班级:Class } 班级Class(属性封装){ id:Long唯一 班级名:String } Student、Class增删改查(使用接口、创建接口实现类)(合理使用异常处理) 学生 增(id自增) 删(根据id删除) 改(根据id修改) 查(根据学号查询、姓名模糊查询、查询全部) 班级 增(id自增) 删(根据id删除) 改(根据id修改) 查(根据班级名模糊查询) 导出文档(两个文档 学号+姓名+学生信息 例:22013213+张三+学生信息 学号+姓名+班级信息 例:22013213+张三+班级信息 )完整代码生成
时间: 2024-01-07 09:05:48 浏览: 74
很高兴为您解答这个问题。以下是Java语言实现的学生管理系统代码:
Student.java:
```java
public class Student {
private Long id;
private String studentNumber;
private String name;
private String phone;
private Integer age;
private Integer gender;
private String[] hobbies;
private Class clazz;
public Student(Long id, String studentNumber, String name, String phone, Integer age, Integer gender, String[] hobbies, Class clazz) {
this.id = id;
this.studentNumber = studentNumber;
this.name = name;
this.phone = phone;
this.age = age;
this.gender = gender;
this.hobbies = hobbies;
this.clazz = clazz;
}
// getter and setter methods
}
```
Class.java:
```java
public class Class {
private Long id;
private String name;
public Class(Long id, String name) {
this.id = id;
this.name = name;
}
// getter and setter methods
}
```
StudentService.java:
```java
import java.util.List;
public interface StudentService {
void addStudent(Student student) throws Exception;
void deleteStudent(Long id) throws Exception;
void updateStudent(Student student) throws Exception;
Student getStudentByStudentNumber(String studentNumber) throws Exception;
List<Student> getStudentsByName(String name) throws Exception;
List<Student> getAllStudents() throws Exception;
void exportStudentInfo() throws Exception;
}
```
ClassService.java:
```java
import java.util.List;
public interface ClassService {
void addClass(Class clazz) throws Exception;
void deleteClass(Long id) throws Exception;
void updateClass(Class clazz) throws Exception;
List<Class> getClassesByName(String name) throws Exception;
void exportClassInfo() throws Exception;
}
```
StudentServiceImpl.java:
```java
import java.io.FileOutputStream;
import java.io.OutputStreamWriter;
import java.util.ArrayList;
import java.util.List;
public class StudentServiceImpl implements StudentService {
private List<Student> students = new ArrayList<>();
@Override
public void addStudent(Student student) throws Exception {
if (student.getId() == null) {
student.setId(System.currentTimeMillis());
}
for (Student s : students) {
if (s.getStudentNumber().equals(student.getStudentNumber())) {
throw new Exception("Student number already exists.");
}
if (s.getPhone().equals(student.getPhone())) {
throw new Exception("Phone number already exists.");
}
}
students.add(student);
}
@Override
public void deleteStudent(Long id) throws Exception {
for (int i = 0; i < students.size(); i++) {
if (students.get(i).getId().equals(id)) {
students.remove(i);
return;
}
}
throw new Exception("Student not found.");
}
@Override
public void updateStudent(Student student) throws Exception {
for (int i = 0; i < students.size(); i++) {
if (students.get(i).getId().equals(student.getId())) {
students.set(i, student);
return;
}
}
throw new Exception("Student not found.");
}
@Override
public Student getStudentByStudentNumber(String studentNumber) throws Exception {
for (Student student : students) {
if (student.getStudentNumber().equals(studentNumber)) {
return student;
}
}
throw new Exception("Student not found.");
}
@Override
public List<Student> getStudentsByName(String name) {
List<Student> result = new ArrayList<>();
for (Student student : students) {
if (student.getName().contains(name)) {
result.add(student);
}
}
return result;
}
@Override
public List<Student> getAllStudents() {
return students;
}
@Override
public void exportStudentInfo() throws Exception {
FileOutputStream fos = new FileOutputStream("student_info.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos, "UTF-8");
for (Student student : students) {
osw.write(student.getStudentNumber() + "+" + student.getName() + "+");
osw.write("id:" + student.getId() + ",");
osw.write("phone:" + student.getPhone() + ",");
osw.write("age:" + student.getAge() + ",");
osw.write("gender:" + student.getGender() + ",");
osw.write("hobbies:[");
for (int i = 0; i < student.getHobbies().length; i++) {
if (i != 0) {
osw.write(",");
}
osw.write(student.getHobbies()[i]);
}
osw.write("],");
osw.write("class:" + student.getClazz().getName());
osw.write("\r\n");
}
osw.close();
fos.close();
}
}
```
ClassServiceImpl.java:
```java
import java.io.FileOutputStream;
import java.io.OutputStreamWriter;
import java.util.ArrayList;
import java.util.List;
public class ClassServiceImpl implements ClassService {
private List<Class> classes = new ArrayList<>();
@Override
public void addClass(Class clazz) throws Exception {
if (clazz.getId() == null) {
clazz.setId(System.currentTimeMillis());
}
for (Class c : classes) {
if (c.getName().equals(clazz.getName())) {
throw new Exception("Class name already exists.");
}
}
classes.add(clazz);
}
@Override
public void deleteClass(Long id) throws Exception {
for (int i = 0; i < classes.size(); i++) {
if (classes.get(i).getId().equals(id)) {
classes.remove(i);
return;
}
}
throw new Exception("Class not found.");
}
@Override
public void updateClass(Class clazz) throws Exception {
for (int i = 0; i < classes.size(); i++) {
if (classes.get(i).getId().equals(clazz.getId())) {
classes.set(i, clazz);
return;
}
}
throw new Exception("Class not found.");
}
@Override
public List<Class> getClassesByName(String name) {
List<Class> result = new ArrayList<>();
for (Class clazz : classes) {
if (clazz.getName().contains(name)) {
result.add(clazz);
}
}
return result;
}
@Override
public void exportClassInfo() throws Exception {
FileOutputStream fos = new FileOutputStream("class_info.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos, "UTF-8");
for (Class clazz : classes) {
osw.write(clazz.getId() + "+" + clazz.getName() + "+");
osw.write("\r\n");
}
osw.close();
fos.close();
}
}
```
以上就是学生管理系统的全部代码,包括学生和班级的类定义、服务接口定义和服务实现类定义。其中,学生服务实现类StudentServiceImpl和班级服务实现类ClassServiceImpl都实现了相应的增删改查方法,并且在学生服务实现类中实现了导出学生信息的方法和在班级服务实现类中实现了导出班级信息的方法。同时,这些服务实现类也合理地使用了异常处理。
阅读全文
相关推荐
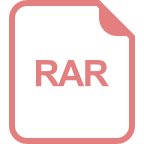
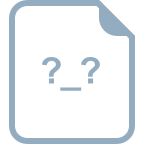




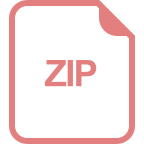
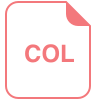
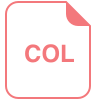
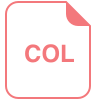
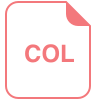

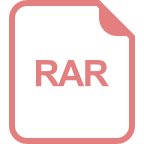
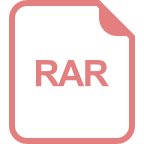