已知一个链表,表中记录按学号递增有序,要求在表中增加一个学生的记录,该学生的学号、姓名、英语成绩、高数成绩、计算机成绩从键盘输入,增加记录后,表仍递增有序。用c语言编写,生成完整代码
时间: 2024-10-15 19:06:15 浏览: 35
为了满足您的需求,我们首先需要创建一个链表结构,然后实现插入新节点并保持链表递增有序的功能。下面是一个简单的C语言示例,它假设您已经有了一个结构体`Student`用于存储学生信息,并有一个链表节点`Node`。这里我们使用头插法(insert at the beginning)来保证链表的递增顺序。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int studentID;
char name[50];
float englishScore;
float mathScore;
float computerScore;
struct Node* next;
} StudentNode;
// 链表头部指针
StudentNode* head = NULL;
void insertStudent() {
// 获取用户输入
int id;
scanf("%d", &id);
char name[50];
fgets(name, sizeof(name), stdin); // 读取名字,忽略回车符
name[strlen(name) - 1] = '\0'; // 去除行尾的换行符
float englishScore, mathScore, computerScore;
scanf("%f %f %f", &englishScore, &mathScore, &computerScore);
// 创建新节点
StudentNode* newNode = (StudentNode*)malloc(sizeof(StudentNode));
newNode->studentID = id;
strncpy(newNode->name, name, sizeof(newNode->name) - 1);
newNode->englishScore = englishScore;
newNode->mathScore = mathScore;
newNode->computerScore = computerScore;
newNode->next = head;
// 插入到链表开始位置,如果当前为空则直接指向新节点
if (head == NULL) {
head = newNode;
} else {
while (head->studentID > id) { // 比较节点值,找到插入位置
head = head->next;
}
newNode->next = head; // 将新节点插入合适的位置
}
}
int main() {
int choice;
do {
printf("请选择操作:\n1. 插入学生\n2. 查看链表\n(其他退出)\n");
scanf("%d", &choice);
switch (choice) {
case 1:
insertStudent();
break;
// ... 其他选项的处理 ...
}
} while (choice != 2); // 如果选择不是2,则继续循环
return 0;
}
```
请注意,这个程序仅实现了基本功能,实际应用中还需要考虑错误处理,如输入验证以及链表遍历查看等。此外,链表的删除和查找等功能可以根据需求进一步扩展。
阅读全文
相关推荐
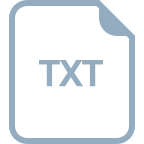
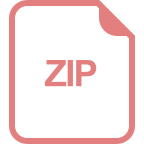
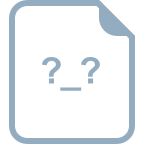
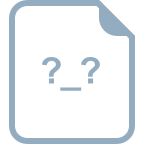
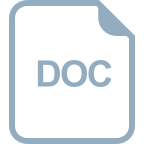
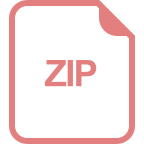
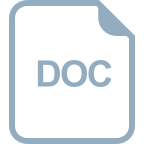
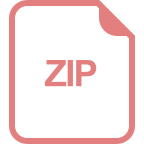
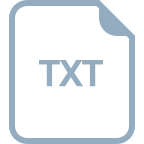
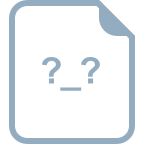
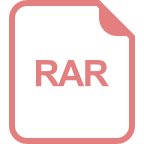
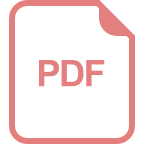