systemverilog dpi改变动态数组大小的程序示例
时间: 2023-08-07 19:01:48 浏览: 257
Certainly! Here's an example of a SystemVerilog DPI program that demonstrates how to dynamically resize an array using DPI:
```systemverilog
// C code
#include <stdio.h>
#include <stdlib.h>
// DPI function declaration
extern "C" int* resize_dynamic_array(int* dynamic_array, int current_size, int new_size);
// SystemVerilog module
module top;
// Dynamic array declaration
int dynamic_array[];
// DPI import statement
import "DPI-C" function int* resize_dynamic_array(int* dynamic_array, int current_size, int new_size);
initial begin
// Initialize the dynamic array with size 5
dynamic_array = new[5];
for (int i = 0; i < 5; i++)
dynamic_array[i] = i;
// Call the DPI function to resize the dynamic array to size 10
dynamic_array = resize_dynamic_array(dynamic_array, 5, 10);
// Print the resized dynamic array
for (int i = 0; i < 10; i++)
$display("Element %d: %d", i, dynamic_array[i]);
// Free the memory allocated for the dynamic array
delete(dynamic_array);
end
endmodule
// DPI implementation in C
int* resize_dynamic_array(int* dynamic_array, int current_size, int new_size) {
int* resized_array = (int*)malloc(new_size * sizeof(int));
for (int i = 0; i < current_size; i++)
resized_array[i] = dynamic_array[i];
free(dynamic_array);
return resized_array;
}
```
In this example, we have a SystemVerilog module `top` that declares a dynamic array `dynamic_array` and imports a DPI function `resize_dynamic_array` from C. The `resize_dynamic_array` function takes in the current dynamic array, its current size, and the new desired size.
In the `initial` block, we initialize the dynamic array with values from 0 to 4. Then, we call the DPI function `resize_dynamic_array` to resize the dynamic array from size 5 to size 10. The DPI function implementation in C allocates memory for the resized array, copies the elements from the old array to the new array, frees the memory of the old array, and returns the resized array.
Finally, we print the resized dynamic array and free the memory allocated for it using the `delete` keyword.
Please note that this example assumes you have a SystemVerilog simulator that supports DPI (Direct Programming Interface) for interfacing with C code. The exact steps to compile and run this code may vary depending on your simulator and toolchain.
阅读全文
相关推荐
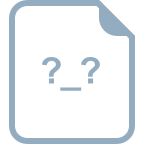
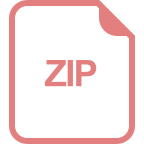


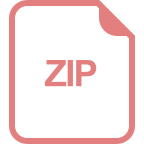
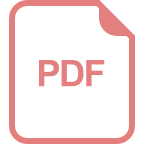
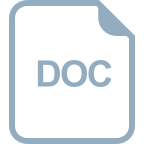
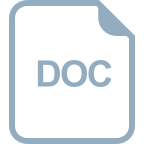
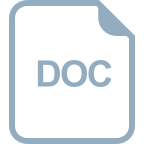



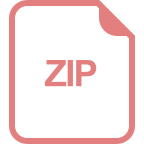
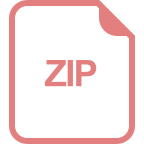
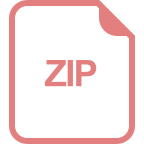