设计一个简单的Python的售卖系统小白
时间: 2024-10-10 08:13:42 浏览: 29
设计一个简单的Python售卖系统的步骤可能会包括以下几个部分:
1. **基本数据结构**:创建两个基础类,如 `Product` 和 `Inventory`。`Product` 类可以包含属性如产品名称、价格和库存等,`Inventory` 则用于存储商品列表。
```python
class Product:
def __init__(self, name, price, stock):
self.name = name
self.price = price
self.stock = stock
class Inventory:
def __init__(self):
self.products = []
```
2. **添加/删除商品**:为 `Inventory` 类添加方法,允许添加新商品 (`add_product`) 和移除商品 (`remove_product`)。
```python
def add_product(self, product):
self.products.append(product)
def remove_product(self, product_name):
for prod in self.products:
if prod.name == product_name:
self.products.remove(prod)
break
```
3. **购买操作**:在 `Inventory` 中添加 `purchase_item` 方法,检查商品库存并更新。
```python
def purchase_item(self, item_name, quantity):
for product in self.products:
if product.name == item_name and product.stock >= quantity:
product.stock -= quantity
print(f"Bought {quantity} of {product.name}, remaining stock: {product.stock}")
return True
print("Item not found or out of stock.")
return False
```
4. **用户交互**:提供一个简单的命令行界面,让用户选择操作(查看库存、购买商品等)。
```python
if __name__ == "__main__":
inventory = Inventory()
while True:
user_input = input("Enter 'add <product> <price> <stock>' to add a product, 'buy <item> <quantity>' to buy, or 'quit' to exit: ")
if user_input.lower() == "quit":
break
# 提供解析和调用相应方法的简单逻辑
# (这里仅作示例,实际应用需要更完善的输入处理)
command, *args = user_input.split()
if command == "add":
inventory.add_product(Product(*args))
elif command == "buy":
inventory.purchase_item(*args)
else:
print("Invalid command.")
```
阅读全文
相关推荐
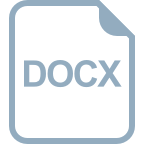
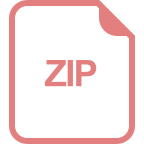
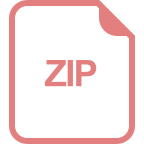

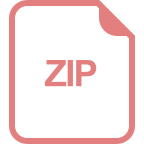
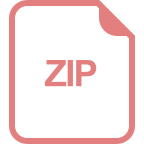
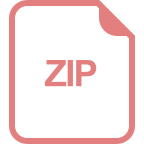
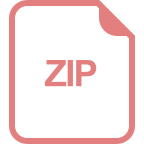
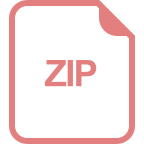
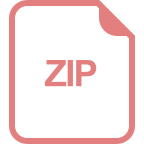
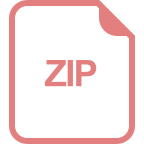
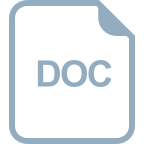
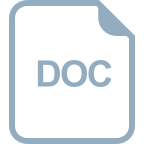
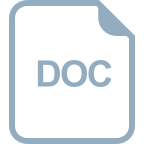

