使用while循环处理列表和字典,python代码
时间: 2023-02-06 17:21:03 浏览: 438
在 Python 中,可以使用 while 循环来遍历列表和字典。
下面是一个示例,展示了如何使用 while 循环遍历列表:
```
# 定义一个列表
my_list = [1, 2, 3, 4, 5]
# 定义索引变量
index = 0
# 开始 while 循环
while index < len(my_list):
# 获取当前元素
item = my_list[index]
print(item)
# 将索引变量加 1
index += 1
```
输出结果如下:
```
1
2
3
4
5
```
下面是一个示例,展示了如何使用 while 循环遍历字典:
```
# 定义一个字典
my_dict = {'a': 1, 'b': 2, 'c': 3}
# 定义一个迭代器
iter = my_dict.items()
# 开始 while 循环
while True:
# 获取下一个键值对
try:
key, value = next(iter)
print(key, value)
except StopIteration:
# 当迭代器用尽时,跳出循环
break
```
输出结果如下:
```
a 1
b 2
c 3
```
注意:上述示例中,我们使用了 try-except 语句来捕获 StopIteration 异常,用于终止 while 循环。在 Python 中,当字典的迭代器用尽时,会抛出 StopIteration 异常。
阅读全文
相关推荐
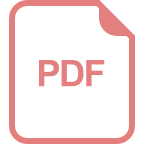
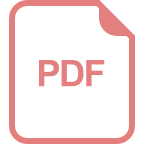
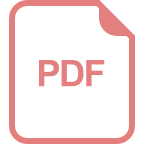
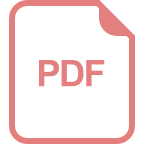
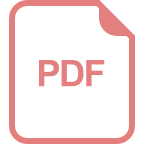
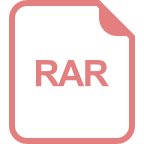
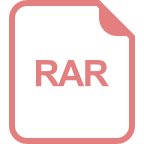
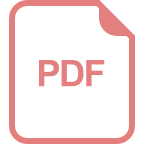
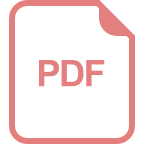
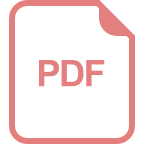
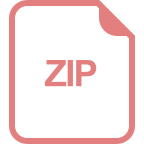
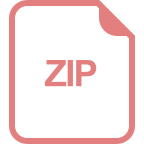
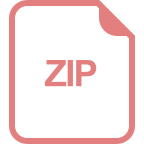
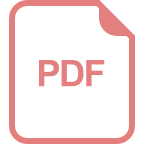
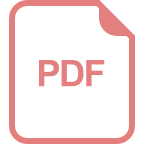
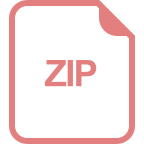
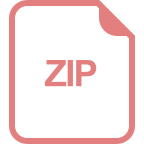