利用C语言或C++使用find函数从指定长度的字符串中查找指定字符串
时间: 2023-08-14 15:06:04 浏览: 215
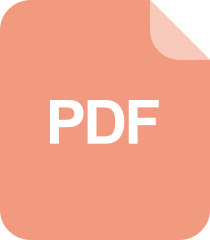
C语言中求字符串长度的函数的几种实现方法

下面是一个用C++实现的例子:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello, world!";
string substr = "world";
int pos = str.find(substr, 0, substr.length());
if (pos != string::npos) {
cout << "Substring found at position " << pos << endl;
} else {
cout << "Substring not found" << endl;
}
return 0;
}
```
在上面的例子中,我们使用了`std::string`类的`find`函数来查找子串。`find`函数的第一个参数是要查找的子串,第二个参数是查找的起始位置,第三个参数是子串的长度。如果找到了子串,`find`函数返回子串在字符串中的位置,否则返回`string::npos`。
如果你想用C语言实现,可以使用`strstr`函数:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "hello, world!";
char substr[] = "world";
char *pos = strstr(str, substr);
if (pos != NULL) {
printf("Substring found at position %ld\n", pos - str);
} else {
printf("Substring not found\n");
}
return 0;
}
```
在上面的例子中,我们使用了`strstr`函数来查找子串。`strstr`函数的第一个参数是要查找的字符串,第二个参数是要查找的子串。如果找到了子串,`strstr`函数返回子串在字符串中的指针,否则返回`NULL`。为了得到子串在字符串中的位置,我们可以用`pos - str`来计算偏移量。
阅读全文
相关推荐
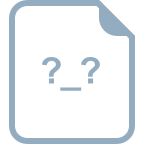
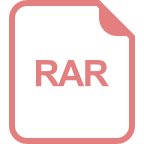
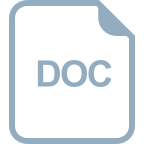
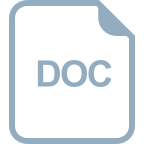
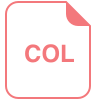









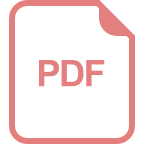