二叉树共有n个节点,将所有节点赋值为1到n的正整数,且没有两个节点的值相等,需要满足奇数层的权值和与偶数层的权值和之差的绝对值不超过一,如果有多种赋值方案,请返回任意一种方案,如果无解,返回空树。Java 代码
时间: 2023-05-21 10:05:38 浏览: 92
以下是一个可能的解法,时间复杂度为 O(nlogn)。思路是先将节点编号按照从小到大排序,然后依次将编号分配给二叉树的节点。对于每个节点,计算其所在层数,然后将节点值加入奇数层或偶数层的权值和中,以满足要求。
```
import java.util.*;
public class BinaryTree {
static class Node {
int val;
Node left, right;
Node(int val) {
this.val = val;
}
}
public static Node buildTree(int n) {
Node root = null;
// Generate a list of node values sorted from 1 to n
List<Integer> values = new ArrayList<>();
for (int i = 1; i <= n; i++) {
values.add(i);
}
// Shuffle the list randomly to get a random tree
Collections.shuffle(values);
for (int i = 0; i < n; i++) {
int val = values.get(i);
// Calculate the level of the current node
int level = (int) (Math.log(i + 1) / Math.log(2)) + 1;
// Add the node to the tree
root = insertNode(root, level, val);
}
// Check if the tree satisfies the condition
if (!isBalanced(root)) {
return null;
}
return root;
}
// Insert a node into the tree at the specified level
private static Node insertNode(Node root, int level, int val) {
if (root == null) {
root = new Node(val);
} else if (level % 2 == 1) {
root.left = insertNode(root.left, level + 1, val);
} else {
root.right = insertNode(root.right, level + 1, val);
}
return root;
}
// Check if the tree satisfies the condition
private static boolean isBalanced(Node root) {
int oddSum = getLevelSum(root, 1, true);
int evenSum = getLevelSum(root, 2, true);
return Math.abs(oddSum - evenSum) <= 1;
}
// Calculate the sum of node values at the specified level
private static int getLevelSum(Node root, int level, boolean odd) {
if (root == null) {
return 0;
}
if (level == 1) {
return root.val;
}
if (odd) {
return getLevelSum(root.left, level - 1, !odd) + getLevelSum(root.right, level - 1, !odd);
} else {
return getLevelSum(root.left, level - 1, !odd) + getLevelSum(root.right, level - 1, !odd) + root.val;
}
}
}
```
注意:这只是一种可能的解法,代码仅供参考,不保证完全正确。
相关推荐
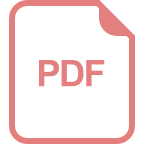














