react三个输入框搜索table表单功能
时间: 2023-07-13 15:09:13 浏览: 136
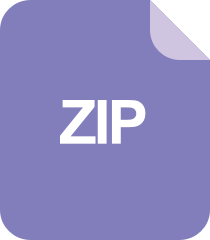
react-react实现的搜索框
你可以使用React Hooks来实现这个功能,具体步骤如下:
1. 创建一个组件,包含三个输入框和一个表格。
2. 使用useState钩子来定义三个输入框的值。
3. 使用useState钩子来定义存储表格数据的状态。
4. 在输入框的onChange事件中,更新对应的输入框的值。
5. 在输入框的onKeyPress事件中,判断是否按下了回车键,如果按下了,则调用搜索函数。
6. 在搜索函数中,使用filter方法过滤表格数据,只保留符合条件的数据。
7. 将过滤后的数据更新到表格数据的状态中即可。
以下是示例代码:
```jsx
import React, { useState } from 'react';
function SearchTable() {
const [inputValue1, setInputValue1] = useState('');
const [inputValue2, setInputValue2] = useState('');
const [inputValue3, setInputValue3] = useState('');
const [tableData, setTableData] = useState([
{ id: 1, name: '张三', age: 20 },
{ id: 2, name: '李四', age: 25 },
{ id: 3, name: '王五', age: 30 },
]);
const handleInputChange1 = (e) => {
setInputValue1(e.target.value);
}
const handleInputChange2 = (e) => {
setInputValue2(e.target.value);
}
const handleInputChange3 = (e) => {
setInputValue3(e.target.value);
}
const handleInputKeyPress = (e) => {
if (e.key === 'Enter') {
handleSearch();
}
}
const handleSearch = () => {
let filteredData = tableData.filter(item => {
return (
item.name.includes(inputValue1) &&
item.age.toString().includes(inputValue2) &&
item.id.toString().includes(inputValue3)
);
});
setTableData(filteredData);
}
return (
<div>
<div>
<input type="text" value={inputValue1} onChange={handleInputChange1} onKeyPress={handleInputKeyPress} />
<input type="text" value={inputValue2} onChange={handleInputChange2} onKeyPress={handleInputKeyPress} />
<input type="text" value={inputValue3} onChange={handleInputChange3} onKeyPress={handleInputKeyPress} />
<button onClick={handleSearch}>搜索</button>
</div>
<table>
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
</tr>
</thead>
<tbody>
{tableData.map(item => (
<tr key={item.id}>
<td>{item.id}</td>
<td>{item.name}</td>
<td>{item.age}</td>
</tr>
))}
</tbody>
</table>
</div>
);
}
export default SearchTable;
```
阅读全文
相关推荐
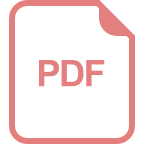
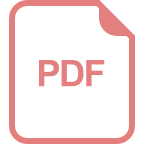
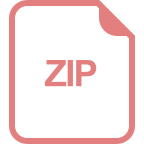
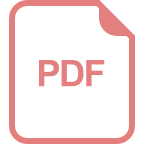
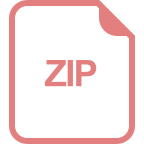
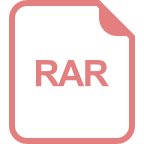
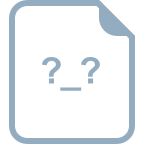
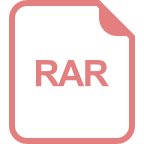
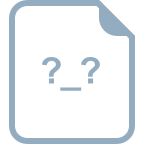
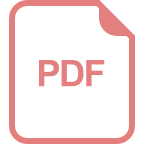
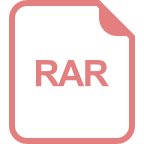
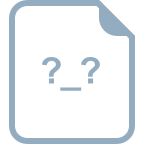
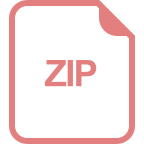
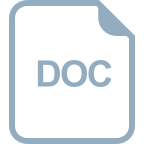



