编写一个函数: char *ReplaceString(char * str, char * s1, char * s2); 该函数用s2替换str中的s1,函数返回替换后的字符串的指针。 题目中已经给出main函数,main函数会调用ReplaceString函数进行测试,依次输入字符串str、s1和s2,最后输出替换后字符串。
时间: 2024-02-28 12:56:34 浏览: 140
好的,下面是代码实现:
```C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char *ReplaceString(char *str, char *s1, char *s2);
int main()
{
char str[100], s1[20], s2[20];
printf("请输入原始字符串:\n");
fgets(str, 100, stdin);
str[strlen(str) - 1] = '\0'; // 去掉fgets读入的换行符
printf("请输入要替换的子串:\n");
fgets(s1, 20, stdin);
s1[strlen(s1) - 1] = '\0';
printf("请输入替换后的子串:\n");
fgets(s2, 20, stdin);
s2[strlen(s2) - 1] = '\0';
char *result = ReplaceString(str, s1, s2);
printf("替换后的字符串为:%s\n", result);
free(result); // 释放动态分配的内存
return 0;
}
char *ReplaceString(char *str, char *s1, char *s2)
{
int len1 = strlen(s1);
int len2 = strlen(s2);
char *result = (char *)malloc(strlen(str) + 1); // 分配足够的内存存储替换后的字符串
int i = 0, j = 0;
while (str[i] != '\0')
{
if (strncmp(str + i, s1, len1) == 0) // 找到要替换的子串
{
strncpy(result + j, s2, len2); // 替换为s2
i += len1;
j += len2;
}
else // 不需要替换,直接复制
{
result[j] = str[i];
i++;
j++;
}
}
result[j] = '\0'; // 注意要在末尾加上'\0'
return result;
}
```
首先在`main`函数中读入原始字符串`str`、要替换的子串`s1`和替换后的子串`s2`,然后调用`ReplaceString`函数进行替换,并输出替换后的字符串。
`ReplaceString`函数中,首先计算出`s1`和`s2`的长度,然后动态分配足够的内存存储替换后的字符串。接着使用两个指针`i`和`j`分别遍历原始字符串和替换后的字符串,如果找到要替换的子串`s1`,就将其替换为`s2`;否则直接复制到替换后的字符串中。最后记得在末尾加上`\0`。最后返回替换后的字符串的指针。
阅读全文
相关推荐
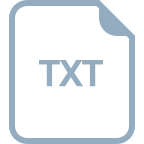
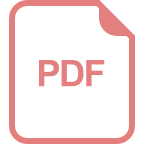










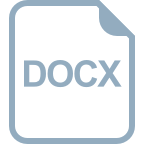
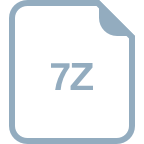