springboot freemarker 自定义列表循环标签 带网页标签的示例
时间: 2023-08-31 19:18:57 浏览: 50
### 回答1:
下面是一个示例,展示如何在Spring Boot中使用FreeMarker自定义列表循环标签,并带有网页标签:
1. 自定义标签类
```java
package com.example.demo.freemarker.tags;
import java.io.IOException;
import java.util.List;
import java.util.Map;
import org.springframework.stereotype.Component;
import freemarker.core.Environment;
import freemarker.template.SimpleScalar;
import freemarker.template.TemplateDirectiveBody;
import freemarker.template.TemplateDirectiveModel;
import freemarker.template.TemplateException;
import freemarker.template.TemplateModel;
import freemarker.template.TemplateModelException;
@Component
public class CustomListTag implements TemplateDirectiveModel {
@Override
public void execute(Environment env, Map params, TemplateModel[] loopVars, TemplateDirectiveBody body)
throws TemplateException, IOException {
// 获取参数
String data = params.get("data").toString();
String item = params.get("item").toString();
// 获取列表数据
List list = (List) env.getVariable(data).getAsList();
// 循环处理
for (int i = 0; i < list.size(); i++) {
// 设置循环变量
env.setVariable(item, new SimpleScalar(list.get(i).toString()));
// 处理标签体
if (body != null) {
body.render(env.getOut());
}
}
}
}
```
2. 页面示例
```html
<#list items as item>
<tr>
<td>${item.name}</td>
<td>${item.price}</td>
<td>${item.description}</td>
</tr>
</#list>
<!-- 使用自定义标签 -->
<@customListTag data="items" item="item">
<tr>
<td>${item.name}</td>
<td>${item.price}</td>
<td>${item.description}</td>
</tr>
</@customListTag>
```
在上面的示例中,我们定义了一个自定义标签类 CustomListTag,它可以接收两个参数:data 和 item。data 参数指定列表数据的变量名,item 参数指定循环变量的名称。
在页面中,我们使用了 FreeMarker 内置的列表循环标签,同时也使用了我们自定义的标签 customListTag。注意,我们在自定义标签中使用了一个标签体,它会在循环中被重复渲染。
### 回答2:
在Spring Boot中使用Freemarker模板引擎时,我们可以自定义列表循环标签,并且在循环中嵌套网页标签来实现一些特定功能。下面以一个简单的示例展示如何实现。
假设我们有一个列表数据,每个数据包含姓名和年龄属性。我们希望在模板中展示这个列表,并使用自定义的循环标签将姓名和年龄分别显示为h1和h2标签。
首先,我们需要创建一个类来实现自定义的循环标签逻辑,命名为CustomListDirective。在该类中,我们需要继承spring提供的AbstractTemplateDirectiveModel,并实现其中的execute方法,该方法用于处理自定义标签的逻辑。
```java
public class CustomListDirective extends AbstractTemplateDirectiveModel {
@Override
public void execute(Environment env, Map params, TemplateModel[] loopVars, TemplateDirectiveBody body) throws TemplateException, IOException {
if (loopVars.length != 1) {
throw new TemplateModelException("This directive requires exactly one loop variable.");
}
List<Map<String, String>> list = (List<Map<String, String>>) params.get("list");
if (list != null) {
for (int i = 0; i < list.size(); i++) {
Map<String, String> item = list.get(i);
loopVars[0] = new SimpleHash(item);
if (body != null) {
body.render(env.getOut());
}
}
}
}
}
```
然后,在Freemarker模板中,我们需要添加自定义标签的命名空间和使用方式。我们在模板中通过自定义标签的名字`customList`来调用我们刚刚创建的CustomListDirective。
```html
<#assign custom = customListDirective />
<#list userList as user>
<@custom.list list=user>
<h1>${user.name}</h1>
<h2>${user.age}</h2>
</@custom.list>
</#list>
```
最后,我们需要将自定义标签的类注册到Freemarker模板引擎中。在Spring Boot的配置文件中,我们可以通过配置`freemarkerSettings`来实现。
```java
@Configuration
public class FreemarkerConfig {
@Autowired
private Configuration configuration;
@PostConstruct
public void setSharedVariable() {
configuration.setSharedVariable("customListDirective", new CustomListDirective());
}
}
```
通过以上步骤,我们就实现了自定义列表循环标签,并且在循环中嵌套了网页标签的示例。当我们在模板中使用`<#list>`指令遍历列表时,将会根据自定义标签的逻辑将姓名和年龄分别插入到h1和h2标签中。
### 回答3:
Spring Boot是一种快速开发框架,而FreeMarker是一款流行的模板引擎。在Spring Boot中使用FreeMarker可以方便地进行动态页面生成。如果我们想要自定义一个列表循环标签,并在其中使用网页标签,可以按照以下步骤进行操作。
首先,我们需要在FreeMarker的配置文件中定义自定义指令或宏。可以通过在`spring.ftl`(默认配置文件)中添加如下代码:
```
<#macro customLoop list>
<ul>
<#list list as item>
<li>${item}</li>
</#list>
</ul>
</#macro>
```
接下来,在我们的模板文件中使用这个自定义指令或宏。假设我们有一个数据模型`model`,其中包含一个名为`listData`的列表,我们可以在模板中这样使用:
```
<@customLoop list=model.listData />
```
这样就可以生成一个带有网页标签的列表。当模板被渲染时,`customLoop`指令会被调用,并在页面上生成一个有序列表。每个列表项都带有`<li>`标签,并显示了`listData`列表中的每个元素。
在上述示例中,我们通过自定义指令的方式实现了一个简单的列表循环标签,并且可以灵活地在其中嵌入网页标签。通过这种方式,我们可以更方便地进行页面的生成和定制。当然,这只是一个简单的示例,实际应用中可能会涉及更复杂的逻辑和操作。
需要注意的是,以上代码只是简要示例,实际开发中可能需要根据具体需求进行适当的调整和扩展。
相关推荐
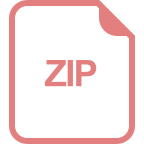













