用c++定义一个学生类Student和教师类Teacher,学生类有姓名、学号,教师类有姓名、工作证号、职称、课程、周学时数。再定义一个助教类TA,继承学生类和教师类,该类可以使用学生类的全部数据成员,以及教师类的课程和周学
时间: 2024-04-23 18:05:06 浏览: 207
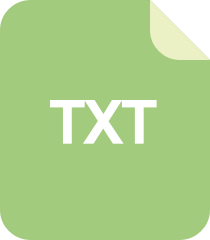
人員信息管理,教師,學生,c++
时数数据成员。以下是用C++定义的代码:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string name;
string id;
public:
Student(string n, string i) : name(n), id(i) {}
string getName() { return name; }
string getId() { return id; }
};
class Teacher {
private:
string name;
string workId;
string title;
string course;
int weeklyHours;
public:
Teacher(string n, string w, string t, string c, int h) : name(n), workId(w), title(t), course(c), weeklyHours(h) {}
string getName() { return name; }
string getWorkId() { return workId; }
string getTitle() { return title; }
string getCourse() { return course; }
int getWeeklyHours() { return weeklyHours; }
};
class TA : public Student, public Teacher {
public:
TA(string n, string i, string w, string t, string c, int h) : Student(n, i), Teacher(n, w, t, c, h) {}
};
int main() {
TA ta("Tom", "S1001", "W1002", "Assistant Professor", "C++ Programming", 10);
cout << "TA Name: " << ta.getName() << endl;
cout << "TA ID: " << ta.getId() << endl;
cout << "TA Work ID: " << ta.getWorkId() << endl;
cout << "TA Title: " << ta.getTitle() << endl;
cout << "TA Course: " << ta.getCourse() << endl;
cout << "TA Weekly Hours: " << ta.getWeeklyHours() << endl;
return 0;
}
```
上述代码定义了三个类Student、Teacher和TA,其中TA类继承了Student和Teacher类。在main函数中,我们创建了一个TA对象,并使用对象的成员函数获取相关数据成员的值,并输出到屏幕上。
阅读全文
相关推荐
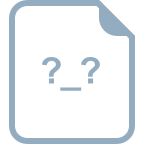


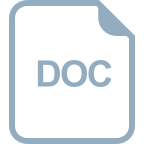
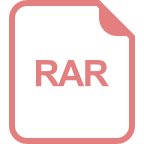
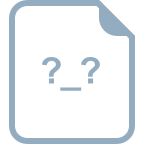
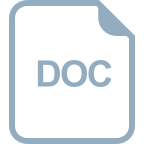
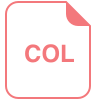

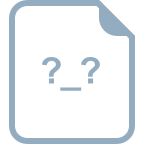
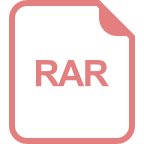
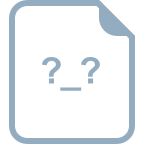
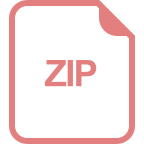