androidstudio用按钮四人中随机抽取两人
时间: 2024-03-01 13:52:27 浏览: 57
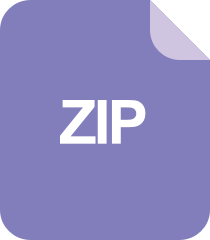
Android Studio中,将两个项目合并到一个项目中
可以使用以下代码实现:
```java
// 定义四个人的名字
String[] names = {"张三", "李四", "王五", "赵六"};
// 生成两个随机数,表示选出的两个人的下标
Random random = new Random();
int index1 = random.nextInt(4);
int index2;
do {
index2 = random.nextInt(4);
} while (index2 == index1);
// 获取选出的两个人的名字
String selected1 = names[index1];
String selected2 = names[index2];
```
这段代码首先定义了一个包含四个人名字的字符串数组,然后使用 `java.util.Random` 类生成两个随机数,表示选出的两个人的下标。由于不能选出同一个人,所以在选出第二个人的时候需要用 `do-while` 循环来保证两个下标不相同。最后,根据选出的两个下标获取对应的人名。
阅读全文
相关推荐
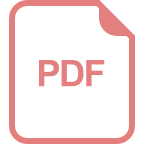
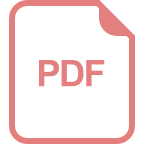



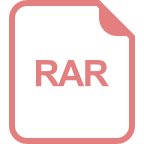
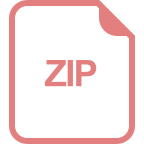
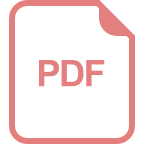
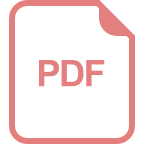
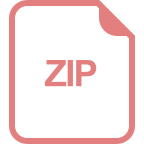
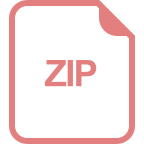
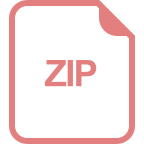
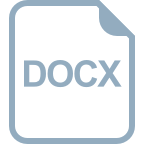
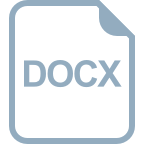