文档要求写出Android需要实现的功能并写出实现代码
时间: 2024-12-18 22:22:42 浏览: 7
### 实验功能需求与实现代码
#### 功能需求
1. **使用服务的方式实现应用程序之间的通信**:通过 Android 中的 AIDL (Android Interface Definition Language) 实现进程间的通信。
2. **创建 AIDL 文件**:定义带有方法签名的编程接口。
3. **实现接口**:扩展 `Stub` 类并实现 AIDL 接口中定义的方法。
4. **向客户端公开接口**:实现 `Service` 并重写 `onBind()` 方法,返回 `Stub` 类的实现。
5. **客户端和服务端交互**:客户端通过绑定服务获取服务端提供的数据(如学号和姓名),并在界面上显示。
#### 实现代码
##### 1. 创建 AIDL 文件
在服务端项目中创建一个 AIDL 文件,假设文件名为 `IStudentService.aidl`:
```aidl
// IStudentService.aidl
package com.example.service;
interface IStudentService {
String getStudentInfo();
}
```
##### 2. 实现接口
在服务端项目中创建一个 `StudentService` 类,实现 `IStudentService.Stub`:
```java
// StudentService.java
package com.example.service;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.os.RemoteException;
public class StudentService extends Service {
private final IStudentService.Stub binder = new IStudentService.Stub() {
@Override
public String getStudentInfo() throws RemoteException {
return "学号: 123456, 姓名: 张三";
}
};
@Override
public IBinder onBind(Intent intent) {
return binder;
}
}
```
##### 3. 配置 `AndroidManifest.xml`
在服务端项目的 `AndroidManifest.xml` 中注册 `StudentService`:
```xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.service">
<application
android:allowBackup="true"
android:label="@string/app_name">
<service android:name=".StudentService">
<intent-filter>
<action android:name="com.example.service.IStudentService" />
</intent-filter>
</service>
</application>
</manifest>
```
##### 4. 客户端实现
在客户端项目中创建一个 AIDL 文件夹,并复制服务端的 `IStudentService.aidl` 文件到该文件夹中。
在客户端项目的 `MainActivity` 中编写逻辑代码,绑定服务并获取学生信息:
```java
// MainActivity.java
package com.example.client;
import android.content.ComponentName;
import android.content.Context;
import android.content.Intent;
import android.content.ServiceConnection;
import android.os.Bundle;
import android.os.IBinder;
import android.os.RemoteException;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import com.example.service.IStudentService;
public class MainActivity extends AppCompatActivity {
private TextView textView;
private IStudentService studentService;
private boolean isBound = false;
private ServiceConnection connection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
studentService = IStudentService.Stub.asInterface(service);
try {
String info = studentService.getStudentInfo();
textView.setText(info);
} catch (RemoteException e) {
e.printStackTrace();
}
}
@Override
public void onServiceDisconnected(ComponentName name) {
studentService = null;
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textView = findViewById(R.id.textView);
Intent intent = new Intent();
intent.setComponent(new ComponentName("com.example.service", "com.example.service.StudentService"));
bindService(intent, connection, Context.BIND_AUTO_CREATE);
isBound = true;
}
@Override
protected void onDestroy() {
super.onDestroy();
if (isBound) {
unbindService(connection);
isBound = false;
}
}
}
```
##### 5. 配置客户端的 `AndroidManifest.xml`
在客户端项目的 `AndroidManifest.xml` 中添加必要的权限:
```xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.client">
<application
android:allowBackup="true"
android:label="@string/app_name">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
```
### 总结
通过上述步骤,我们实现了使用 AIDL 在 Android 应用程序之间进行进程间通信的功能。服务端提供了一个 `StudentService` 服务,客户端通过绑定该服务获取学生信息并在界面上显示。
阅读全文
相关推荐
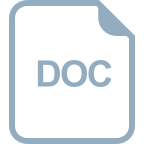
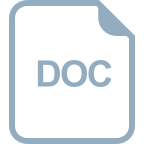
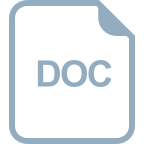
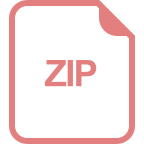
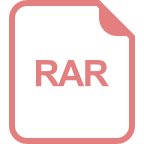
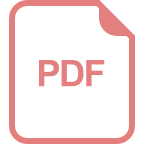
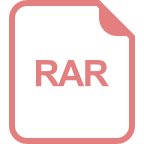











