C和c++批量遍历多个文件夹内文件,要注释
时间: 2024-02-04 18:03:06 浏览: 30
您好,可以使用以下步骤进行批量遍历多个文件夹内文件并注释:
1. 使用递归方式遍历文件夹内的所有文件。
2. 对于每个文件,判断其文件类型是否为C或C++文件,可以使用文件后缀名进行判断。
3. 对于C或C++文件,使用文件输入输出流读取文件内容,将需要注释的代码行进行注释处理,可以使用正则表达式或字符串处理方式进行注释。
4. 使用文件输入输出流将处理后的文件内容写入原文件或写入新的文件中。
下面是一个C++示例代码,可以实现上述功能:
```cpp
#include <iostream>
#include <fstream>
#include <regex>
#include <filesystem>
using namespace std;
namespace fs = std::filesystem;
void commentFile(const string& filename)
{
string line;
string content;
ifstream inputFile(filename);
if (!inputFile.is_open())
{
cout << "Failed to open file: " << filename << endl;
return;
}
regex pattern("\\s*(//|/\\*|\\*/|#).*");
while (getline(inputFile, line))
{
if (regex_match(line, pattern))
{
content += line + "\n";
}
else
{
content += "// " + line + "\n";
}
}
inputFile.close();
ofstream outputFile(filename);
if (!outputFile.is_open())
{
cout << "Failed to open file: " << filename << endl;
return;
}
outputFile << content;
outputFile.close();
}
void commentFolder(const string& path)
{
for (const auto& entry : fs::recursive_directory_iterator(path))
{
if (entry.is_regular_file())
{
const string& filename = entry.path().string();
if (filename.substr(filename.find_last_of(".") + 1) == "c"
|| filename.substr(filename.find_last_of(".") + 1) == "cpp")
{
commentFile(filename);
}
}
}
}
int main()
{
commentFolder("C:/MyProject");
return 0;
}
```
这段代码遍历指定文件夹内的所有C和C++文件,并对文件内容进行注释处理,处理后的文件内容将覆盖原文件。您可以根据需要进行修改,比如将处理后的文件写入新的文件中,而不是覆盖原文件。
相关推荐
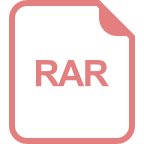
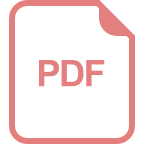
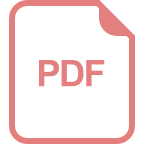














