MATLAB configureCallback
时间: 2025-01-03 15:12:30 浏览: 9
### 如何在MATLAB中配置回调函数
在MATLAB中,回调函数用于响应特定事件的发生。这些事件可以是由用户操作触发的(如按钮点击),也可以是程序运行过程中发生的其他类型的事件。
#### 创建简单的GUI并设置回调函数
为了展示如何配置回调函数,在下面的例子中创建了一个带有按钮的小型图形用户界面(GUI),当按下该按钮时会调用一个自定义的回调函数:
```matlab
function createSimpleGuiWithCallback()
% Create a figure window.
f = uifigure('Name', 'Simple GUI with Callback');
% Add a button to the figure and set its callback property.
b = uibutton(f, 'Text', 'Click Me!', ...
'ButtonPushedFcn', @(src,event)buttonClicked(src,event));
end
% Define the callback function that will be called when the button is clicked.
function buttonClicked(src,event)
disp('The button was clicked!');
end
```
上述代码片段展示了如何在一个UI组件上指定`'ButtonPushedFcn'`属性来关联一个匿名函数作为其回调处理逻辑[^1]。
对于更复杂的场景,比如希望在整个应用程序生命周期内多次重用某个回调方法,则可以把这类方法写成独立于任何具体控件之外的形式,并通过名称传递给相应的事件监听器。
#### 使用句柄类实现持久化的回调环境
有时可能需要让回调访问外部变量或状态信息而不仅仅是简单地打印消息到命令窗口。这时可以通过继承自`handle`基类的对象保存上下文数据以便后续查询更新:
```matlab
classdef PersistentContext < handle
properties
Counter;
end
methods
function obj = PersistentContext()
obj.Counter = 0;
end
function incrementCounter(~, ~, srcObj)
srcObj.Counter = srcObj.Counter + 1;
fprintf('Button has been pressed %d times.\n',...
srcObj.Counter);
end
end
end
function setupPersistentCallbackDemo()
ctx = PersistentContext();
fig = uifigure('Name','Persistent Context Demo');
btn = uibutton(fig,'Text','Increment Counter',...
'Position',[20 20 150 40],...
'ButtonPushedFcn',{@ctx.incrementCounter, ctx});
end
```
在这个例子中,每当用户单击按钮时都会增加计数器的值,并显示当前已累计了多少次点击次数。
阅读全文
相关推荐


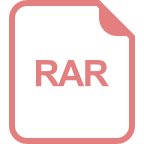
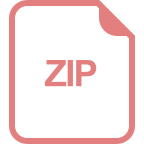
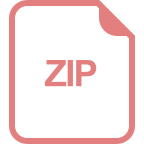
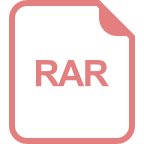
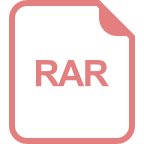

