你能用C语言写stm32使用SPI接口控制AD9851的程序吗
时间: 2023-12-03 14:46:58 浏览: 98
当然可以,以下是一个基本的示例程序:
```c
#include "stm32f10x.h"
#define SPI_PORT SPI1
#define SPI_SCK_PIN GPIO_Pin_5
#define SPI_SCK_GPIO_PORT GPIOA
#define SPI_MISO_PIN GPIO_Pin_6
#define SPI_MISO_GPIO_PORT GPIOA
#define SPI_MOSI_PIN GPIO_Pin_7
#define SPI_MOSI_GPIO_PORT GPIOA
#define AD9851_CS_PIN GPIO_Pin_4
#define AD9851_CS_GPIO_PORT GPIOA
void SPI_Configuration(void);
uint8_t SPI_SendByte(uint8_t byte);
void AD9851_Init(void);
void AD9851_SetFrequency(uint32_t frequency);
int main (void)
{
SystemInit();
SPI_Configuration();
AD9851_Init();
AD9851_SetFrequency(1000000); // Set frequency to 1MHz
while(1)
{
// Do something
}
}
void SPI_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
SPI_InitTypeDef SPI_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SPI1 | RCC_APB2Periph_GPIOA, ENABLE);
/* Configure SPI pins */
GPIO_InitStructure.GPIO_Pin = SPI_SCK_PIN | SPI_MOSI_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(SPI_SCK_GPIO_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = SPI_MISO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(SPI_MISO_GPIO_PORT, &GPIO_InitStructure);
/* Configure AD9851 CS pin */
GPIO_InitStructure.GPIO_Pin = AD9851_CS_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(AD9851_CS_GPIO_PORT, &GPIO_InitStructure);
/* Configure SPI */
SPI_InitStructure.SPI_Direction = SPI_Direction_1Line_Tx;
SPI_InitStructure.SPI_Mode = SPI_Mode_Master;
SPI_InitStructure.SPI_DataSize = SPI_DataSize_8b;
SPI_InitStructure.SPI_CPOL = SPI_CPOL_Low;
SPI_InitStructure.SPI_CPHA = SPI_CPHA_1Edge;
SPI_InitStructure.SPI_NSS = SPI_NSS_Soft;
SPI_InitStructure.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_4;
SPI_InitStructure.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_Init(SPI_PORT, &SPI_InitStructure);
SPI_Cmd(SPI_PORT, ENABLE);
}
uint8_t SPI_SendByte(uint8_t byte)
{
/* Wait for the SPI transmit buffer to be empty */
while (SPI_I2S_GetFlagStatus(SPI_PORT, SPI_I2S_FLAG_TXE) == RESET);
/* Send the byte through the SPI peripheral */
SPI_I2S_SendData(SPI_PORT, byte);
/* Wait for the SPI receive buffer to be full */
while (SPI_I2S_GetFlagStatus(SPI_PORT, SPI_I2S_FLAG_RXNE) == RESET);
/* Return the received byte */
return SPI_I2S_ReceiveData(SPI_PORT);
}
void AD9851_Init(void)
{
/* Set the AD9851 to sleep mode */
GPIO_ResetBits(AD9851_CS_GPIO_PORT, AD9851_CS_PIN);
SPI_SendByte(0x00);
SPI_SendByte(0x04);
SPI_SendByte(0x00);
SPI_SendByte(0x00);
GPIO_SetBits(AD9851_CS_GPIO_PORT, AD9851_CS_PIN);
}
void AD9851_SetFrequency(uint32_t frequency)
{
uint32_t freq_word = frequency * 4294967296.0 / 125000000.0;
/* Set the AD9851 to frequency mode */
GPIO_ResetBits(AD9851_CS_GPIO_PORT, AD9851_CS_PIN);
SPI_SendByte(0x00);
SPI_SendByte(0x02);
SPI_SendByte((freq_word >> 24) & 0xFF);
SPI_SendByte((freq_word >> 16) & 0xFF);
SPI_SendByte((freq_word >> 8) & 0xFF);
SPI_SendByte(freq_word & 0xFF);
GPIO_SetBits(AD9851_CS_GPIO_PORT, AD9851_CS_PIN);
}
```
这个程序使用的是 STM32F1 系列的芯片,并且使用 SPI1 接口与 AD9851 芯片进行通信。SPI_SendByte 函数用于发送一个字节的数据,AD9851_Init 函数用于初始化 AD9851 芯片,AD9851_SetFrequency 函数用于设置 AD9851 的频率。注意,这个程序中的频率计算公式是针对 125MHz 的时钟频率的,如果使用的时钟频率不同,则需要相应地调整此公式。
阅读全文
相关推荐
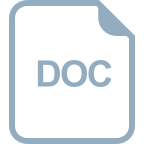
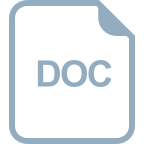
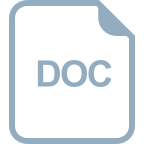
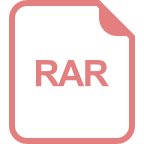
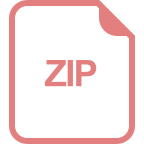
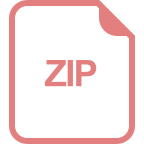
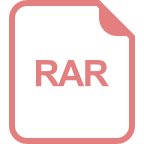
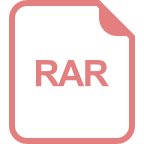
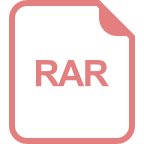
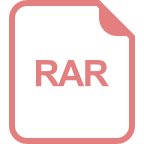
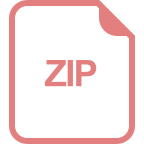


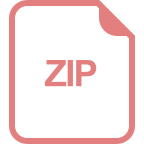
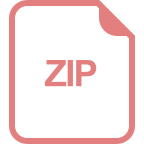
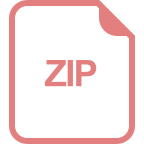
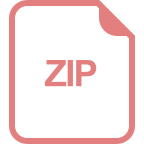
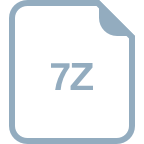
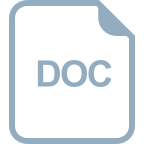