C# 多线程 打印 奇偶数
时间: 2023-11-26 20:38:04 浏览: 32
使用AutoResetEvent实现多线程打印奇偶数的方法如下:
```csharp
static AutoResetEvent oddEvent = new AutoResetEvent(false);
static AutoResetEvent evenEvent = new AutoResetEvent(false);
static int count = 1;
static void Main(string[] args)
{
Thread t1 = new Thread(PrintOddNumbers);
Thread t2 = new Thread(PrintEvenNumbers); t1.Start();
t2.Start();
t1.Join();
t2.Join();
Console.ReadLine();
}
static void PrintOddNumbers()
{
while (count <= 100)
{
if (count % 2 == 1)
{
Console.WriteLine("Thread 1: " + count);
count++;
evenEvent.Set();
oddEvent.WaitOne();
}
}
}
static void PrintEvenNumbers()
{
while (count <= 100)
{
if (count % 2 == 0)
{
Console.WriteLine("Thread 2: " + count);
count++;
oddEvent.Set();
evenEvent.WaitOne();
}
}
}
```
另一种使用Monitor实现多线程打印奇偶数的方法如下:
```csharp
static object lockObj = new object();
static int count = 1;
static void Main(string[] args)
{
Thread t1 = new Thread(PrintOddNumbers);
Thread t2 = new Thread(PrintEvenNumbers);
t1.Start();
t2.Start();
t1.Join();
t2.Join();
Console.ReadLine();
}
static void PrintOddNumbers()
{
while (count <= 100)
{
lock (lockObj)
{
if (count % 2 == 1)
{
Console.WriteLine("Thread 1: " + count);
count++;
}
}
}
}
static void PrintEvenNumbers()
{
while (count <= 100)
{
lock (lockObj)
{
if (count % 2 == 0)
{
Console.WriteLine("Thread 2: " + count);
count++;
}
}
}
}
```
相关推荐
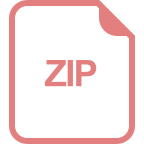














