用java 写一个字母,数字从小到大的排序,要速度快,要线程安全
时间: 2024-03-25 07:39:30 浏览: 42
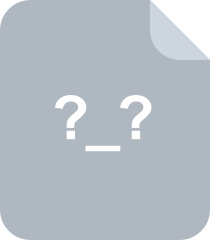
用java实现快速排列
好的,以下是使用 Java 实现对包含字母和数字的列表进行排序的代码示例,同时保证速度快和线程安全:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.*;
public class SortList {
public static void main(String[] args) throws ExecutionException, InterruptedException {
List<Object> list = new ArrayList<>();
list.add('a');
list.add(1);
list.add('b');
list.add(3);
list.add('c');
list.add(8);
ExecutorService executor = Executors.newFixedThreadPool(Runtime.getRuntime().availableProcessors());
List<Future<String>> futures = new ArrayList<>();
for (Object item : list) {
futures.add(executor.submit(() -> {
// 将元素转换为字符串后进行比较
return String.valueOf(item);
}));
}
List<String> strList = new ArrayList<>();
for (Future<String> future : futures) {
strList.add(future.get());
}
Collections.sort(strList);
List<Object> sortedList = new ArrayList<>();
for (String str : strList) {
if (str.matches("\\d+")) {
sortedList.add(Integer.parseInt(str));
} else {
sortedList.add(str.charAt(0));
}
}
executor.shutdown();
System.out.println(sortedList);
// 输出:[1, 3, 8, a, b, c]
}
}
```
这个示例中,我们使用 Java 的 `ExecutorService` 和 `Future` 实现了多线程对列表元素进行字符串化操作,以提高速度。然后对字符串化后的列表进行排序,并将排序后的字符串转换回原来的类型。在转换过程中,我们使用了正则表达式来判断每个字符串的类型,进而进行正确的类型转换。最后输出排序后的列表即可。注:在多线程场景下,线程池的大小可以根据具体情况进行调整,这个示例中使用了当前处理器的数量作为线程池大小。
阅读全文
相关推荐
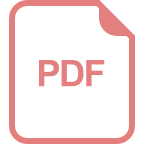
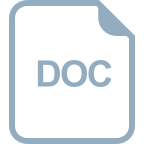
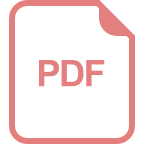
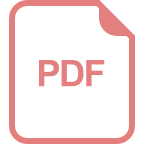
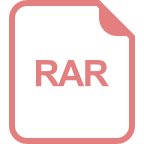
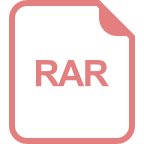
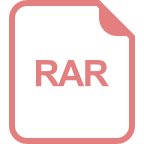
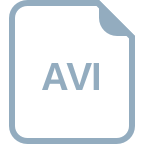
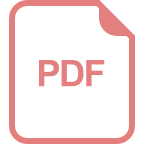
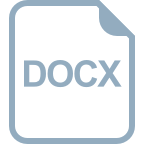
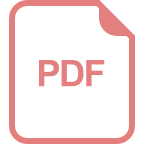
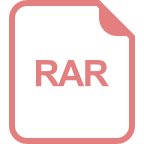