C# Alert弹出框自动关闭
时间: 2023-07-12 08:15:56 浏览: 189
你可以使用JavaScript来实现自动关闭弹出框。以下是一个例子:
```javascript
<script type="text/javascript">
function showAlert() {
alert("这是一个弹出框。");
setTimeout(function () {
$('.alert').hide();
}, 5000);
}
</script>
```
在这个例子中,`showAlert`函数会弹出一个警告框。然后使用`setTimeout`函数将弹出框隐藏在5秒钟后。
你也可以使用`setInterval`函数来定期检查弹出框是否需要关闭。例如:
```javascript
<script type="text/javascript">
function showAlert() {
alert("这是一个弹出框。");
setInterval(function () {
if ($('.alert').is(":visible")) {
$('.alert').hide();
}
}, 5000);
}
</script>
```
在这个例子中,`setInterval`函数将每5秒钟检查一次弹出框是否可见。如果可见,它会将其隐藏。
相关问题
C# Alert弹出框自动关闭实例
以下是一个 C# Alert 弹出框自动关闭的示例:
```csharp
using System;
using System.Windows.Forms;
using System.Threading;
public class Program
{
static void Main()
{
// 创建一个 Alert 弹出框
var alert = new Alert("这是一个自动关闭的弹出框", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
// 启动一个新线程,等待 3 秒后关闭弹出框
new Thread(() =>
{
Thread.Sleep(3000);
alert.Invoke(new Action(() => alert.Close()));
}).Start();
// 显示 Alert 弹出框
alert.ShowDialog();
}
}
public class Alert : Form
{
public Alert(string message, string caption, MessageBoxButtons buttons, MessageBoxIcon icon)
{
Text = caption;
FormBorderStyle = FormBorderStyle.FixedDialog;
MaximizeBox = false;
MinimizeBox = false;
ShowInTaskbar = false;
StartPosition = FormStartPosition.CenterScreen;
Icon = SystemIcons.Information;
ControlBox = false;
var label = new Label
{
Text = message,
AutoSize = true,
Location = new System.Drawing.Point(10, 10)
};
Controls.Add(label);
var okButton = new Button
{
DialogResult = DialogResult.OK,
Text = "确定",
Location = new System.Drawing.Point(140, 60)
};
Controls.Add(okButton);
AcceptButton = okButton;
DialogResult = DialogResult.OK;
}
}
```
这个示例创建了一个 Alert 弹出框,并在启动一个新线程后等待 3 秒后自动关闭弹出框。你可以根据需要修改线程等待时间。
C# web Alert弹出框自动关闭实例
以下是一个在 Web 应用程序中实现自动关闭 Alert 弹出框的示例:
ASPX 页面代码:
```aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="WebApplication1.Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>自动关闭 Alert 弹出框示例</title>
<script type="text/javascript">
function autoCloseAlert() {
window.setTimeout(function () {
window.location.href = window.location.href;
}, 3000);
}
</script>
</head>
<body onload="autoCloseAlert()">
<form id="form1" runat="server">
<div>
<asp:Button ID="btnShowAlert" runat="server" Text="显示 Alert 弹出框" OnClick="btnShowAlert_Click" />
</div>
</form>
</body>
</html>
```
ASPX.cs 代码:
```csharp
using System;
using System.Web.UI;
namespace WebApplication1
{
public partial class Default : Page
{
protected void btnShowAlert_Click(object sender, EventArgs e)
{
ClientScript.RegisterStartupScript(this.GetType(), "autoCloseAlert", "alert('这是一个自动关闭的 Alert 弹出框');", true);
}
}
}
```
在这个示例中,我们在 ASPX 页面中添加了一个 JavaScript 函数 `autoCloseAlert()`,用于在页面加载完成后等待 3 秒后刷新当前页面,从而关闭 Alert 弹出框。在按钮的事件处理方法中,我们使用 `ClientScript.RegisterStartupScript()` 方法在客户端注册一个脚本,用于显示 Alert 弹出框。
注意:自动关闭的实现是通过刷新页面来实现的,因此在关闭弹出框时可能会出现页面闪烁的情况。
阅读全文
相关推荐










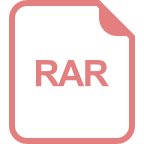


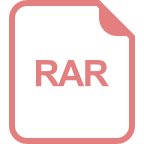


