vue3 ts封装websocket单点登录
时间: 2023-07-02 21:23:01 浏览: 156
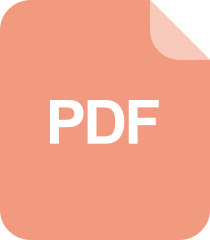
vue+ts下对axios的封装实现
好的,关于Vue3和TypeScript封装Websocket单点登录,我可以给您提供一些思路和代码示例。
首先,我们需要安装`socket.io-client`库,用于在Vue3中建立和管理Websocket连接。可以通过以下命令进行安装:
```
npm install socket.io-client
```
然后,我们可以在Vue3中创建一个`WebSocketService`类,用于管理Websocket连接和消息的发送和接收。以下是一个示例代码:
```typescript
import { io, Socket } from "socket.io-client";
export class WebSocketService {
private static instance: WebSocketService;
private socket: Socket | undefined;
private constructor() {}
public static getInstance(): WebSocketService {
if (!WebSocketService.instance) {
WebSocketService.instance = new WebSocketService();
}
return WebSocketService.instance;
}
public connect(url: string, token: string): void {
this.socket = io(url, {
auth: { token },
});
this.socket.on("connect", () => {
console.log("Websocket connected!");
});
this.socket.on("disconnect", () => {
console.log("Websocket disconnected!");
});
}
public send(event: string, data: any): void {
if (this.socket) {
this.socket.emit(event, data);
} else {
console.error("Websocket not connected!");
}
}
public on(event: string, callback: (data: any) => void): void {
if (this.socket) {
this.socket.on(event, callback);
} else {
console.error("Websocket not connected!");
}
}
public off(event: string, callback: (data: any) => void): void {
if (this.socket) {
this.socket.off(event, callback);
} else {
console.error("Websocket not connected!");
}
}
public disconnect(): void {
if (this.socket) {
this.socket.disconnect();
} else {
console.error("Websocket not connected!");
}
}
}
```
这个类使用了单例模式,确保我们只创建一个Websocket连接。`connect`方法用于建立连接并验证用户身份,`send`方法用于发送消息,`on`方法用于监听消息,`off`方法用于取消监听,`disconnect`方法用于断开连接。
在Vue3组件中,我们可以使用`setup`函数创建一个`WebSocketService`实例,并在需要的时候调用它的方法。以下是一个示例代码:
```typescript
import { defineComponent, onMounted } from "vue";
import { WebSocketService } from "./WebSocketService";
export default defineComponent({
name: "WebSocketDemo",
setup() {
const webSocketService = WebSocketService.getInstance();
onMounted(() => {
const url = "ws://localhost:3000";
const token = "mytoken";
webSocketService.connect(url, token);
});
webSocketService.on("message", (data) => {
console.log("Received message:", data);
});
const sendMessage = () => {
const data = { text: "Hello, world!" };
webSocketService.send("message", data);
};
return {
sendMessage,
};
},
});
```
在这个组件中,我们在`onMounted`钩子中调用`connect`方法建立连接。然后,我们使用`on`方法监听`message`事件,并定义了一个`sendMessage`方法用于发送消息。
这就是一个简单的Vue3和TypeScript封装Websocket单点登录的示例。希望对您有所帮助!
阅读全文
相关推荐
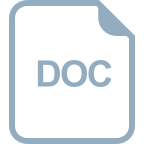
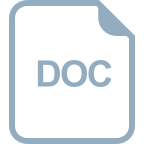

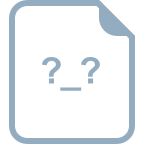
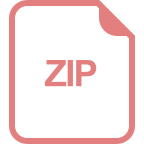
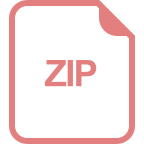
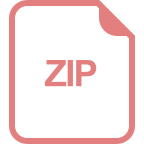
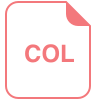



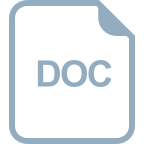
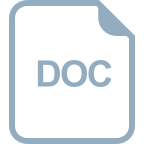
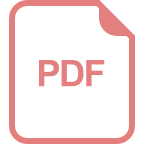
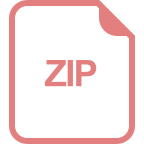
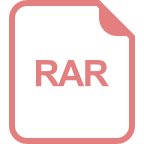
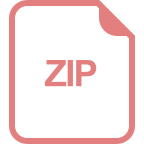
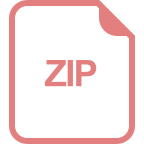