用vhdl编写一个关于carMP3的红外信号接收器代码
时间: 2023-09-06 11:07:37 浏览: 105
好的,下面是一个简单的红外信号接收器的 VHDL 代码,它可以接收 carMP3 发送的红外信号,解码后将命令输出到一个输出端口。请注意,这只是一个简单的示例代码,具体实现还需要根据你的具体需求进行修改和调整。
```
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity ir_receiver is
port (
clk: in std_logic;
ir_input: in std_logic;
ir_decoded: out std_logic_vector(7 downto 0)
);
end entity ir_receiver;
architecture rtl of ir_receiver is
type state_type is (idle, start, data);
signal state: state_type;
signal data_reg: std_logic_vector(31 downto 0);
signal data_reg_next: std_logic_vector(31 downto 0);
signal bit_count: unsigned(3 downto 0);
signal bit_count_next: unsigned(3 downto 0);
begin
ir_decoder: process(clk)
begin
if rising_edge(clk) then
if state = idle then
if ir_input = '0' then
state <= start;
end if;
elsif state = start then
if ir_input = '1' then
state <= data;
bit_count <= (others => '0');
data_reg <= (others => '0');
end if;
elsif state = data then
if ir_input = '1' then
bit_count_next <= bit_count + 1;
data_reg_next <= data_reg(30 downto 0) & '1';
elsif bit_count = 16 then
ir_decoded <= data_reg(23 downto 16);
state <= idle;
else
bit_count_next <= bit_count;
data_reg_next <= data_reg(30 downto 0) & '0';
end if;
end if;
end if;
end process ir_decoder;
bit_counter: process(clk)
begin
if rising_edge(clk) then
bit_count <= bit_count_next;
data_reg <= data_reg_next;
end if;
end process bit_counter;
end architecture rtl;
```
在这个代码中,我们定义了一个状态机,它有三个状态:`idle`,`start`和`data`。一旦检测到红外输入信号,状态机就会从 `idle` 转移到 `start` 状态,并等待一个完整的起始位信号。一旦检测到起始位信号,状态机就会进入 `data` 状态,开始接收数据位。在接收到16个数据位后,状态机会将解码后的命令输出到 `ir_decoded` 端口,并返回 `idle` 状态。
请注意,这个代码只是一个简单的示例,实际使用时可能需要根据具体的红外协议进行修改。同时,还需要根据具体的硬件平台进行适当的修改和调整。
阅读全文
相关推荐
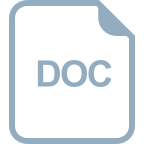
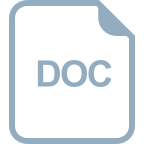
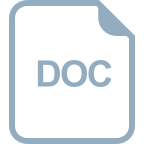
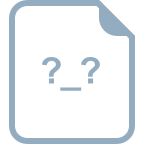
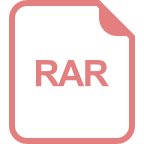
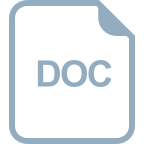
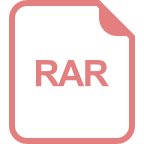
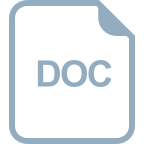
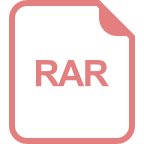
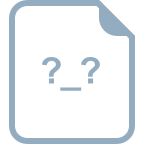
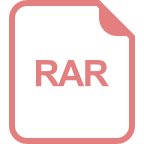
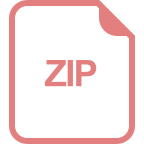
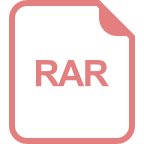