void CSingleDocTestDoc::Serialize(CArchive& ar) { if (ar.IsStoring()) { // TODO: 在此添加存储代码 ar << m_CurrentData; } else { // TODO: 在此添加加载代码 ar >> m_CurrentData; } }
时间: 2024-04-11 11:33:22 浏览: 12
您的 `Serialize` 函数在保存和加载时都正确地处理了 `m_CurrentData` 字符串数据。在保存时,将 `m_CurrentData` 写入存档 (`CArchive`),在加载时,从存档中读取 `m_CurrentData`。
在上述代码的 "// TODO" 注释下添加存储和加载代码是您需要实现的部分。您可以根据需要在这些位置添加其他操作。
例如,在保存代码部分,您可以将其他需要保存的数据写入存档。在加载代码部分,您可以从存档中读取其他需要加载的数据。
以下是一个示例,展示了如何在 `Serialize` 函数中存储和加载其他数据:
```cpp
void CSingleDocTestDoc::Serialize(CArchive& ar)
{
if (ar.IsStoring())
{
// 存储 m_CurrentData
ar << m_CurrentData;
// 存储其他数据
ar << m_OtherData;
}
else
{
// 加载 m_CurrentData
ar >> m_CurrentData;
// 加载其他数据
ar >> m_OtherData;
}
}
```
在上述示例中,`m_OtherData` 是另一个需要存储和加载的数据成员。您可以根据需要添加更多的存储和加载代码。
希望这能帮助到您!
相关问题
int m_nData[10];数据保存),此项工作需要在虚函数Serialize(CArchive& ar)中完成。
在虚函数Serialize(CArchive& ar)中完成数据的保存,可以按照以下步骤进行:
1. 首先需要判断是进行数据的保存还是加载,可以通过CArchive对象的IsStoring()函数来判断,如果返回值为TRUE,则表示进行数据的保存,否则为加载。
2. 如果是进行数据的保存,可以调用CArchive对象的Write函数将数据写入到存档文件中;如果是进行数据的加载,可以调用CArchive对象的Read函数将数据从存档文件中读取出来。
3. 在具体的实现中,可以使用循环结构遍历m_nData数组中的每一个元素,然后进行数据的写入或读取操作。
下面是一个示例代码:
```
void CMyClass::Serialize(CArchive& ar)
{
if (ar.IsStoring())
{
for (int i = 0; i < 10; i++)
{
ar << m_nData[i];
}
}
else
{
for (int i = 0; i < 10; i++)
{
ar >> m_nData[i];
}
}
}
```
Serialize(CArchive& ar)函数作用
Serialize(CArchive& ar)函数是MFC框架中的一个成员函数,用于将对象的数据序列化到存档文件(archive)或从存档文件中反序列化对象的数据。
具体来说,Serialize函数有两个参数,第一个参数是CArchive对象的引用,用于指定要进行序列化或反序列化的存档文件。第二个参数是一个布尔值,用于指示是进行序列化操作还是反序列化操作。
在序列化过程中,Serialize函数负责将对象的数据以二进制形式写入存档文件中,以便在之后的反序列化过程中能够重新恢复对象的状态。在反序列化过程中,Serialize函数会从存档文件中读取二进制数据,并将其转换为对象的状态。
通过实现Serialize函数,可以使得对象能够在不同的运行环境中进行数据的持久化存储和恢复,方便数据的传输和共享。同时,Serialize函数也是MFC框架中实现对象持久化的重要手段之一。
相关推荐
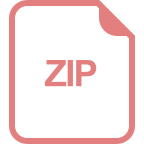
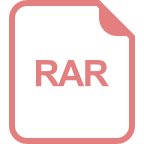
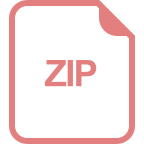












