RANSAC算法拟合平面
时间: 2023-10-27 09:47:45 浏览: 199
RANSAC(RANdom SAmple Consensus)算法是一种经典的随机抽样一致算法,常用于拟合具有噪声的数据。在拟合平面的情况下,RANSAC算法可以通过随机选择数据中的点来估算平面的参数,并根据选取的点是否符合拟合的模型来更新最佳拟合模型。具体步骤如下:
1. 随机选择一定数量的点来构建初始平面模型;
2. 计算平面模型与所有数据点之间的距离,并将距离小于一定阈值的点标记为“内点”;
3. 如果内点数目大于一定阈值,重新估算平面模型;
4. 重复步骤2和3,若达到预设的最大迭代次数或者内点数目满足一定条件,则停止迭代,输出最佳拟合模型。
在拟合平面时,常用的模型表示方法是:
Ax + By + Cz + D = 0
其中,A、B、C表示平面的法向量,D为平面与原点的距离。通过最小二乘法可以求得平面参数的解析解,但是在存在噪声的情况下,解析解可能会受到噪声的影响而偏离真实值。因此,使用RANSAC算法可以更好地处理噪声数据。
相关问题
RANSAC算法拟合平面matlab程序
以下是使用RANSAC算法拟合平面的Matlab程序示例:
```matlab
% 生成数据
n = 200; % 数据点数量
inliers_ratio = 0.7; % 内点比例
noise = 0.1; % 噪声
X = randn(n, 3); % 随机生成点
% 生成一个平面
plane_normal = randn(3,1);
plane_normal = plane_normal./norm(plane_normal);
plane_offset = randn(1);
d = abs(X*plane_normal - plane_offset) < noise; % 标记内点
% 添加一些噪声
d = d & (rand(n,1) < inliers_ratio);
% RANSAC参数
max_iters = 1000;
inlier_threshold = 0.1;
% RANSAC
best_model = [];
best_score = 0;
for iter = 1:max_iters
% 选择随机样本
sample_indices = randperm(n, 3);
sample_points = X(sample_indices, :);
% 计算平面方程
plane_normal = cross(sample_points(2,:)-sample_points(1,:), sample_points(3,:)-sample_points(1,:));
plane_normal = plane_normal./norm(plane_normal);
plane_offset = -plane_normal*sample_points(1,:)';
% 计算内点数量
dists = abs(X*plane_normal - plane_offset);
inliers = dists < inlier_threshold;
score = sum(inliers);
% 更新最好的模型
if score > best_score
best_model = [plane_normal', plane_offset];
best_score = score;
end
end
% 输出结果
disp(['平面法向量:' num2str(best_model(1:3)')]);
disp(['平面偏移量:' num2str(best_model(4))]);
% 绘制结果
figure;
scatter3(X(:,1), X(:,2), X(:,3), 20, d, 'filled');
hold on;
[x,y] = meshgrid(-3:0.1:3, -3:0.1:3);
z = (-best_model(1)*x - best_model(2)*y - best_model(4))/best_model(3);
surf(x,y,z);
axis equal;
```
在本示例中,我们生成200个随机点,并在其中生成一个平面。然后我们添加一些噪声,并使用RANSAC算法拟合平面。最终,我们输出最佳模型的法向量和偏移量,并在图形中绘制结果。
RANSAC算法拟合圆柱,这部分代码细化一下具体步骤并细化
好的,下面是对RANSAC算法拟合圆柱的具体步骤进行细化:
1. 导入必要的库和模块:
```python
import open3d as o3d
import numpy as np
```
2. 加载点云数据:
```python
point_cloud = o3d.io.read_point_cloud("path_to_point_cloud.pcd") # 替换为你的点云文件路径
```
3. 对点云进行平滑处理(可选):
```python
point_cloud_filtered = point_cloud.voxel_down_sample(voxel_size=0.01) # 使用0.01作为体素大小,可根据需要调整
```
4. 定义RANSAC算法参数:
```python
distance_threshold = 0.01 # 平面模型的距离阈值
ransac_n = 3 # RANSAC算法采样的点数
num_iterations = 1000 # 迭代次数
```
5. 定义RANSAC算法函数:
```python
def ransac_cylinder(point_cloud, distance_threshold, ransac_n, num_iterations):
best_cylinder_model = None
best_inlier_indices = []
best_inlier_cloud = None
best_num_inliers = 0
for i in range(num_iterations):
# 随机采样ransac_n个点
sample_indices = np.random.choice(len(point_cloud.points), size=ransac_n, replace=False)
sample_cloud = point_cloud.select_down_sample(sample_indices)
# 拟合圆柱模型
_, _, cylinder_model = sample_cloud.segment_cylinder(radius=0.1, method=o3d.geometry.CylinderModel.FROM_SURFACE_NORMAL)
# 计算所有点到模型的距离
distances = sample_cloud.compute_point_cloud_distance(cylinder_model)
# 计算内点索引
inlier_indices = np.where(distances < distance_threshold)[0]
num_inliers = len(inlier_indices)
# 更新最佳拟合结果
if num_inliers > best_num_inliers:
best_num_inliers = num_inliers
best_inlier_indices = inlier_indices
best_inlier_cloud = sample_cloud.select_down_sample(inlier_indices)
best_cylinder_model = cylinder_model
return best_cylinder_model, best_inlier_indices, best_inlier_cloud
```
6. 调用RANSAC算法函数进行拟合:
```python
cylinder_model, inlier_indices, inlier_cloud = ransac_cylinder(point_cloud_filtered, distance_threshold, ransac_n, num_iterations)
```
7. 获取拟合结果:
```python
cylinder_radius = cylinder_model.radius
```
现在,`cylinder_radius` 变量中存储了拟合出的圆柱半径。请根据实际情况调整参数和处理过程。
阅读全文
相关推荐
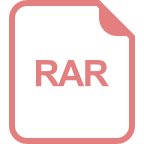
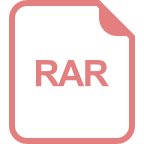
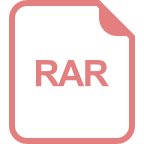
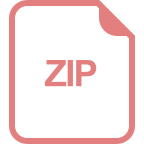
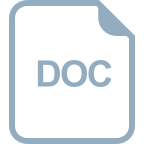
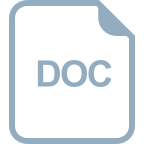







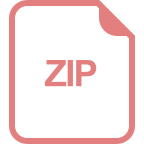