public String SM4encryptCBC(String data) { if (StringUtils.isEmpty(data)) { return ""; } sm4 = new SM4Utils(secretKey.trim(), iv.trim()); return sm4.encryptData_CBC(data); }换成JDK11的类
时间: 2024-01-23 11:01:41 浏览: 112
JDK11中没有内置SM4加密算法的类库,需要使用第三方库或自行实现。可以考虑使用Bouncy Castle库来实现SM4加密。
使用Bouncy Castle库的示例代码如下:
```
import org.bouncycastle.crypto.BlockCipher;
import org.bouncycastle.crypto.BufferedBlockCipher;
import org.bouncycastle.crypto.engines.SM4Engine;
import org.bouncycastle.crypto.modes.CBCBlockCipher;
import org.bouncycastle.crypto.paddings.PaddedBufferedBlockCipher;
import org.bouncycastle.crypto.params.KeyParameter;
import org.bouncycastle.crypto.params.ParametersWithIV;
import java.nio.charset.StandardCharsets;
import java.security.SecureRandom;
import java.util.Base64;
public class SM4Utils {
private static final int BLOCK_SIZE = 16;
private byte[] keyBytes;
private byte[] ivBytes;
public SM4Utils(String key, String iv) {
this.keyBytes = key.getBytes(StandardCharsets.UTF_8);
this.ivBytes = iv.getBytes(StandardCharsets.UTF_8);
}
public String encryptData_CBC(String data) {
try {
byte[] rawData = data.getBytes(StandardCharsets.UTF_8);
// Generate a random IV
byte[] iv = new byte[BLOCK_SIZE];
new SecureRandom().nextBytes(iv);
// Initialize the SM4 cipher in CBC mode with padding
BlockCipher cipher = new SM4Engine();
BufferedBlockCipher cipherWithPadding = new PaddedBufferedBlockCipher(new CBCBlockCipher(cipher));
// Set the key and IV
cipherWithPadding.init(true, new ParametersWithIV(new KeyParameter(keyBytes), ivBytes));
// Encrypt the data
byte[] encryptedData = new byte[cipherWithPadding.getOutputSize(rawData.length)];
int numBytesProcessed = cipherWithPadding.processBytes(rawData, 0, rawData.length, encryptedData, 0);
cipherWithPadding.doFinal(encryptedData, numBytesProcessed);
// Combine the IV and encrypted data
byte[] combinedData = new byte[iv.length + encryptedData.length];
System.arraycopy(iv, 0, combinedData, 0, iv.length);
System.arraycopy(encryptedData, 0, combinedData, iv.length, encryptedData.length);
// Return the encrypted data as a Base64-encoded string
return Base64.getEncoder().encodeToString(combinedData);
} catch (Exception e) {
e.printStackTrace();
return "";
}
}
}
```
你可以使用这段代码来替换原来使用的SM4Utils。注意,这段代码仅供参考,需要根据你的具体需求进行修改和优化。
阅读全文
相关推荐
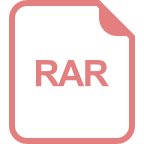
















