用Qt实现计算器的复数的加减乘除
时间: 2024-03-09 13:45:31 浏览: 104
好的,我会尽力回答您的问题。首先,我们需要定义一个复数类。下面是一个简单的复数类定义:
```c++
class ComplexNumber {
public:
ComplexNumber(double r = 0, double i = 0) : real(r), imag(i) {}
ComplexNumber operator+(const ComplexNumber& other) const {
return ComplexNumber(real + other.real, imag + other.imag);
}
ComplexNumber operator-(const ComplexNumber& other) const {
return ComplexNumber(real - other.real, imag - other.imag);
}
ComplexNumber operator*(const ComplexNumber& other) const {
return ComplexNumber(real * other.real - imag * other.imag, real * other.imag + imag * other.real);
}
ComplexNumber operator/(const ComplexNumber& other) const {
double denominator = other.real * other.real + other.imag * other.imag;
return ComplexNumber((real * other.real + imag * other.imag) / denominator, (imag * other.real - real * other.imag) / denominator);
}
double real;
double imag;
};
```
接下来,我们可以使用Qt的UI框架来创建一个简单的计算器界面。我们需要添加一些按钮,如数字按钮、操作符按钮等。当用户按下这些按钮时,我们可以使用复数类来计算结果并在界面上显示结果。下面是一个简单的Qt计算器界面的示例代码:
```c++
#include <QApplication>
#include <QWidget>
#include <QGridLayout>
#include <QLineEdit>
#include <QPushButton>
#include "complexnumber.h"
class Calculator : public QWidget {
public:
Calculator(QWidget* parent = nullptr) : QWidget(parent) {
QGridLayout* layout = new QGridLayout(this);
// 添加显示结果的文本框
QLineEdit* resultLineEdit = new QLineEdit(this);
resultLineEdit->setReadOnly(true);
layout->addWidget(resultLineEdit, 0, 0, 1, 4);
// 添加数字按钮
QPushButton* button0 = new QPushButton("0", this);
layout->addWidget(button0, 4, 0);
connect(button0, &QPushButton::clicked, this, [=]() {
inputDigit("0");
});
// 添加操作符按钮
QPushButton* addButton = new QPushButton("+", this);
layout->addWidget(addButton, 1, 3);
connect(addButton, &QPushButton::clicked, this, [=]() {
operationButtonClicked("+");
});
QPushButton* subtractButton = new QPushButton("-", this);
layout->addWidget(subtractButton, 2, 3);
connect(subtractButton, &QPushButton::clicked, this, [=]() {
operationButtonClicked("-");
});
QPushButton* multiplyButton = new QPushButton("*", this);
layout->addWidget(multiplyButton, 3, 3);
connect(multiplyButton, &QPushButton::clicked, this, [=]() {
operationButtonClicked("*");
});
QPushButton* divideButton = new QPushButton("/", this);
layout->addWidget(divideButton, 4, 3);
connect(divideButton, &QPushButton::clicked, this, [=]() {
operationButtonClicked("/");
});
// 添加清空按钮
QPushButton* clearButton = new QPushButton("C", this);
layout->addWidget(clearButton, 0, 3);
connect(clearButton, &QPushButton::clicked, this, [=]() {
clear();
});
// 添加数字按钮
QPushButton* button1 = new QPushButton("1", this);
layout->addWidget(button1, 3, 0);
connect(button1, &QPushButton::clicked, this, [=]() {
inputDigit("1");
});
QPushButton* button2 = new QPushButton("2", this);
layout->addWidget(button2, 3, 1);
connect(button2, &QPushButton::clicked, this, [=]() {
inputDigit("2");
});
QPushButton* button3 = new QPushButton("3", this);
layout->addWidget(button3, 3, 2);
connect(button3, &QPushButton::clicked, this, [=]() {
inputDigit("3");
});
QPushButton* button4 = new QPushButton("4", this);
layout->addWidget(button4, 2, 0);
connect(button4, &QPushButton::clicked, this, [=]() {
inputDigit("4");
});
QPushButton* button5 = new QPushButton("5", this);
layout->addWidget(button5, 2, 1);
connect(button5, &QPushButton::clicked, this, [=]() {
inputDigit("5");
});
QPushButton* button6 = new QPushButton("6", this);
layout->addWidget(button6, 2, 2);
connect(button6, &QPushButton::clicked, this, [=]() {
inputDigit("6");
});
QPushButton* button7 = new QPushButton("7", this);
layout->addWidget(button7, 1, 0);
connect(button7, &QPushButton::clicked, this, [=]() {
inputDigit("7");
});
QPushButton* button8 = new QPushButton("8", this);
layout->addWidget(button8, 1, 1);
connect(button8, &QPushButton::clicked, this, [=]() {
inputDigit("8");
});
QPushButton* button9 = new QPushButton("9", this);
layout->addWidget(button9, 1, 2);
connect(button9, &QPushButton::clicked, this, [=]() {
inputDigit("9");
});
// 设置计算器界面的大小和标题
setFixedSize(250, 200);
setWindowTitle(tr("复数计算器"));
}
private:
// 当用户按下数字按钮时调用此函数
void inputDigit(const QString& digit) {
if (inputText.isEmpty() || inputText == "+" || inputText == "-" || inputText == "*" || inputText == "/") {
inputText += digit;
} else {
inputText[inputText.length() - 1] = digit[0];
}
updateResultLineEdit();
}
// 当用户按下操作符按钮时调用此函数
void operationButtonClicked(const QString& operation) {
if (!inputText.isEmpty() && inputText != "+" && inputText != "-" && inputText != "*" && inputText != "/") {
if (currentOperation.isEmpty()) {
// 如果当前操作符为空,则保存当前操作符和操作数
currentOperation = operation;
currentNumber.real = inputText.toDouble();
inputText.clear();
updateResultLineEdit();
} else {
// 如果当前操作符不为空,则计算结果,并保存新的操作符和操作数
ComplexNumber newNumber(inputText.toDouble(), 0);
ComplexNumber result;
if (currentOperation == "+") {
result = currentNumber + newNumber;
} else if (currentOperation == "-") {
result = currentNumber - newNumber;
} else if (currentOperation == "*") {
result = currentNumber * newNumber;
} else if (currentOperation == "/") {
result = currentNumber / newNumber;
}
currentOperation = operation;
currentNumber = result;
inputText.clear();
updateResultLineEdit();
}
}
}
// 当用户按下清空按钮时调用此函数
void clear() {
inputText.clear();
currentOperation.clear();
currentNumber.real = 0;
currentNumber.imag = 0;
updateResultLineEdit();
}
// 更新显示结果的文本框
void updateResultLineEdit() {
if (inputText.isEmpty()) {
resultLineEdit->setText(QString::number(currentNumber.real) + " + " + QString::number(currentNumber.imag) + "i");
} else {
resultLineEdit->setText(inputText);
}
}
QLineEdit* resultLineEdit; // 显示结果的文本框
ComplexNumber currentNumber; // 当前操作数
QString currentOperation; // 当前操作符
QString inputText; // 用户输入的文本
};
int main(int argc, char* argv[]) {
QApplication app(argc, argv);
Calculator calculator;
calculator.show();
return app.exec();
}
```
上面的代码演示了如何使用复数类来计算用户输入的复数表达式,并在界面上显示结果。当用户按下数字按钮时,我们将数字添加到输入文本中。当用户按下操作符按钮时,我们将当前操作数和操作符保存下来,并计算结果。当用户按下清空按钮时,我们清除所有保存的状态。最后,我们使用Qt的UI框架来创建一个简单的计算器界面,并将上述函数与按钮的click信号连接起来。
阅读全文
相关推荐
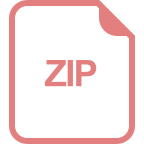
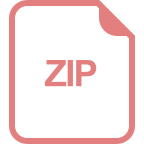
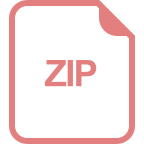















