字符串的循环左移,指的是将字符串整体向左移动,左部超出范围的字符回到右边,比如对于字符串123,它循环左移1位的结果是231。 在右侧编辑器中有一个函数Shift,它有两个参数str和n,str存有
时间: 2024-12-12 15:20:38 浏览: 28
字符串的循环左移操作通常涉及到字符串处理和数组操作。在给定的`Shift`函数中,`str`是你想要移动的原始字符串,而`n`则是移动的位数。这个函数的基本思路通常是创建一个新的字符串,然后将原字符串的每个字符按照指定次数(循环移动`n`次)复制到新位置。如果`n`大于字符串长度,那么会从字符串末尾开始复制。
例如,如果你有一个字符串`"123"`和`n = 2`,首先计算需要移动多少个完整的循环周期(`n`除以字符串长度取余),然后剩下的部分就是直接在新字符串的开头添加。所以:
- 如果余数为0,如`n=3`,只需简单地将字符串拼接到自身即可得到结果:"123123"。
- 如果余数不为0,如`n=4`,则先移动完整周期得到"3121",然后将第一个字符移到末尾得到"2131"。
下面是一个简单的Python示例,展示如何实现这种循环左移:
```python
def Shift(str, n):
length = len(str)
cycles = n // length
remainder = n % length
# 创建新的空字符串用于存放结果
result = ''
# 处理完整的周期
for _ in range(cycles):
result += str
# 处理剩余的字符
if remainder > 0:
result += str[:remainder]
return result
```
相关问题
7-5 LL(1)分析-最左推导输入一个上下文无关文法,先输入文法产生式条数,再输入各个产生式,字符“ε”用“@”表示。 输入一个待分析的字符串,以"#"结束。 输出格式: 输出待分析字符串的最左推导。给我C++代码
好的,以下是一个简单的C++代码实现LL(1)分析器并输出最左推导的例子。请注意,这里的代码实现只是一个简单的示例,如果您想要更加完整和高效的实现,可能需要更多的工作和优化。
```
#include <iostream>
#include <map>
#include <vector>
#include <stack>
using namespace std;
// 定义产生式结构体
struct Production {
char left; // 左部字符
string right; // 右部字符串
};
// 定义LL(1)分析器类
class LL1 {
public:
LL1(int n) : n(n) {
productions.resize(n);
isFirst.resize(256, vector<bool>(false));
isFollow.resize(256, vector<bool>(false));
predictTable.resize(256, vector<int>(256, -1));
}
// 添加产生式
void addProduction(int i, char left, string right) {
productions[i].left = left;
productions[i].right = right;
}
// 计算FIRST集
void calcFirst() {
for (int i = 0; i < n; i++) {
char left = productions[i].left;
string right = productions[i].right;
for (int j = 0; j < right.length(); j++) {
char c = right[j];
if (isupper(c)) { // 非终结符
isFirst[left][c] = true; // FIRST(A) 包含 FIRST(B)
for (int k = 0; k < n; k++) {
if (productions[k].left == c) {
// 如果 B -> ε,则 FIRST(A) 包含 FIRST(B) - {ε}
if (k == i && right.length() == 1) {
isFirst[left][c] = false;
}
for (int l = 0; l < productions[k].right.length(); l++) {
char r = productions[k].right[l];
isFirst[left][r] = true; // FIRST(A) 包含 FIRST(B)
if (!isFirst[c][r]) {
break;
}
}
}
}
} else { // 终结符
isFirst[left][c] = true;
break;
}
}
}
}
// 计算FOLLOW集
void calcFollow() {
isFollow[productions[0].left]['#'] = true;
for (int i = 0; i < n; i++) {
char left = productions[i].left;
string right = productions[i].right;
for (int j = 0; j < right.length(); j++) {
char c = right[j];
if (isupper(c)) { // 非终结符
if (j == right.length() - 1) { // A -> αB
for (int k = 0; k < n; k++) {
if (productions[k].left == left) {
isFollow[c] = isFollow[c] || isFollow[productions[k].right[productions[k].right.length() - 1]];
}
}
} else { // A -> αBβ
char next = right[j + 1];
if (isupper(next)) { // FIRST(β) - {ε} 属于 FOLLOW(B)
for (int k = 0; k < n; k++) {
if (productions[k].left == next) {
for (int l = 0; l < productions[k].right.length(); l++) {
char r = productions[k].right[l];
if (r != '@') {
isFollow[c][r] = true;
}
}
}
}
} else { // β 是终结符,直接加入 FOLLOW(B)
isFollow[c][next] = true;
}
}
}
}
}
}
// 构造预测分析表
void buildPredictTable() {
for (int i = 0; i < n; i++) {
char left = productions[i].left;
string right = productions[i].right;
vector<bool> first = isFirst[left];
for (int j = 0; j < right.length(); j++) {
char c = right[j];
if (isupper(c)) { // 非终结符
for (int k = 0; k < n; k++) {
if (productions[k].left == c) {
for (int l = 0; l < 256; l++) {
if (first[l]) {
predictTable[left][l] = k;
}
}
if (isFirst[c]['@']) { // 如果 B -> ε,则 FOLLOW(B) 属于 FOLLOW(A)
for (int l = 0; l < 256; l++) {
if (isFollow[left][l]) {
predictTable[left][l] = k;
}
}
}
break;
}
}
if (!isFirst[c]['@']) {
break;
}
} else { // 终结符
predictTable[left][c] = i;
break;
}
}
}
}
// LL(1)分析
void analyze(string input) {
stack<char> stk;
stk.push('#');
stk.push(productions[0].left);
int i = 0;
cout << "最左推导: ";
while (!stk.empty()) {
char c = stk.top();
stk.pop();
if (c == input[i]) {
if (c != '#') {
i++;
}
} else if (isupper(c)) {
int prod = predictTable[c][input[i]];
Production p = productions[prod];
cout << p.left << "->" << p.right << " ";
for (int j = p.right.length() - 1; j >= 0; j--) {
if (p.right[j] != '@') {
stk.push(p.right[j]);
}
}
} else {
break;
}
}
cout << endl;
}
private:
int n; // 产生式条数
vector<Production> productions; // 产生式
vector<vector<bool>> isFirst; // FIRST集
vector<vector<bool>> isFollow; // FOLLOW集
vector<vector<int>> predictTable; // 预测分析表
};
int main() {
int n;
cout << "请输入产生式条数: ";
cin >> n;
LL1 ll1(n);
cout << "请输入各个产生式: " << endl;
for (int i = 0; i < n; i++) {
char left;
string right;
cin >> left >> right;
ll1.addProduction(i, left, right);
}
ll1.calcFirst();
ll1.calcFollow();
ll1.buildPredictTable();
string input;
cout << "请输入待分析的字符串: ";
cin >> input;
input += '#';
ll1.analyze(input);
return 0;
}
```
希望这个例子对你有所帮助!
输入文法产生式 2. 计算First集和Follow集 3. 生成预测分析表 4. 输入测试的字符串 5. 求解结果,即判断输入的字符串是否为该文法的句子 采用c++语言,给我代码
好的,下面是一个使用C++语言实现的程序,用于计算文法的First集和Follow集,并生成预测分析表,最后判断输入的字符串是否为该文法的句子。
```cpp
#include <iostream>
#include <vector>
#include <map>
#include <set>
#include <string>
#include <algorithm>
using namespace std;
// 定义产生式结构
struct Production {
string left;
vector<string> right;
};
// 计算First集和Follow集
void calculateFirstAndFollow(const vector<Production>& productions, map<string, set<string>>& firstSets, map<string, set<string>>& followSets) {
// 初始化First集和Follow集
for (const auto& production : productions) {
firstSets[production.left] = set<string>();
followSets[production.left] = set<string>();
}
bool changed = true;
while (changed) {
changed = false;
for (const auto& production : productions) {
string left = production.left;
const vector<string>& right = production.right;
// 计算First集
set<string> currentFirst;
for (const auto& symbol : right) {
if (isupper(symbol[0])) { // 非终结符
currentFirst.insert(firstSets[symbol].begin(), firstSets[symbol].end());
if (firstSets[symbol].find("ε") == firstSets[symbol].end()) {
break;
}
} else { // 终结符
currentFirst.insert(symbol);
break;
}
}
if (currentFirst.size() > firstSets[left].size()) {
firstSets[left].insert(currentFirst.begin(), currentFirst.end());
changed = true;
}
// 计算Follow集
if (right.size() == 0 || find(right.begin(), right.end(), "ε") != right.end()) {
set<string> currentFollow = followSets[left];
for (auto it = right.rbegin(); it != right.rend(); ++it) {
if (isupper((*it)[0])) {
int beforeSize = followSets[*it].size();
followSets[*it].insert(currentFollow.begin(), currentFollow.end());
if (followSets[*it].size() > beforeSize) {
changed = true;
}
if (firstSets[*it].find("ε") == firstSets[*it].end()) {
break;
}
currentFollow = set<string>();
currentFollow.insert(firstSets[*it].begin(), firstSets[*it].end());
currentFollow.erase("ε");
} else {
currentFollow = set<string>();
currentFollow.insert(symbol);
}
}
}
}
}
}
// 生成预测分析表
void generatePredictiveParsingTable(const vector<Production>& productions, const map<string, set<string>>& firstSets, const map<string, set<string>>& followSets, map<pair<string, string>, vector<string>>& parsingTable) {
for (const auto& production : productions) {
string left = production.left;
const vector<string>& right = production.right;
set<string> symbolsFirst;
for (const auto& symbol : right) {
if (isupper(symbol[0])) {
symbolsFirst.insert(firstSets.at(symbol).begin(), firstSets.at(symbol).end());
if (firstSets.at(symbol).find("ε") == firstSets.at(symbol).end()) {
break;
}
} else {
symbolsFirst.insert(symbol);
break;
}
}
for (const auto& symbol : symbolsFirst) {
if (symbol != "ε") {
parsingTable[{left, symbol}] = right;
} else {
for (const auto& followSymbol : followSets.at(left)) {
parsingTable[{left, followSymbol}] = right;
}
}
}
}
}
int main() {
// 输入产生式
vector<Production> productions;
cout << "请输入产生式的数量: ";
int n;
cin >> n;
for (int i = 0; i < n; ++i) {
Production production;
cout << "请输入第 " << i + 1 << " 个产生式的左部: ";
cin >> production.left;
cout << "请输入第 " << i + 1 << " 个产生式的右部 (用空格分隔): ";
string symbol;
while (cin >> symbol) {
if (symbol == "$") {
break;
}
production.right.push_back(symbol);
}
productions.push_back(production);
}
// 计算First集和Follow集
map<string, set<string>> firstSets, followSets;
calculateFirstAndFollow(productions, firstSets, followSets);
// 生成预测分析表
map<pair<string, string>, vector<string>> parsingTable;
generatePredictiveParsingTable(productions, firstSets, followSets, parsingTable);
// 输入测试的字符串
cout << "请输入测试的字符串 (用空格分隔): ";
string input;
cin >> input;
vector<string> inputSymbols;
string symbol;
stringstream ss(input);
while (ss >> symbol) {
inputSymbols.push_back(symbol);
}
inputSymbols.push_back("$");
// 求解结果
string stack = "$";
string currentSymbol = productions[0].left;
bool success = true;
while (!stack.empty() && success) {
if (currentSymbol == inputSymbols[0]) {
stack.pop_back();
inputSymbols.erase(inputSymbols.begin());
} else if (isupper(currentSymbol[0])) {
auto it = parsingTable.find({currentSymbol, inputSymbols[0]});
if (it != parsingTable.end()) {
stack.pop_back();
for (auto it2 = it->second.rbegin(); it2 != it->second.rend(); ++it2) {
stack += *it2;
}
} else {
success = false;
}
} else {
success = false;
}
currentSymbol = stack.empty() ? "" : stack.back();
}
// 输出结果
if (success && inputSymbols[0] == "$") {
cout << "输入的字符串是该文法的句子" << endl;
} else {
cout << "输入的字符串不是该文法的句子" << endl;
}
return 0;
}
```
阅读全文
相关推荐





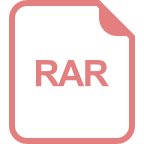






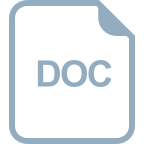



