使用java实现对字符串的aes加密和解密
时间: 2023-06-05 08:47:15 浏览: 195
使用Java实现对字符串的AES加密和解密可以使用Java Cryptography Extension (JCE)提供的API。以下是一个简单的示例代码:
加密:
```
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class AESEncryption {
public static String encrypt(String plainText, String key) throws Exception {
SecretKeySpec secretKeySpec = new SecretKeySpec(key.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec);
byte[] encryptedBytes = cipher.doFinal(plainText.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
}
```
解密:
```
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class AESDecryption {
public static String decrypt(String encryptedText, String key) throws Exception {
SecretKeySpec secretKeySpec = new SecretKeySpec(key.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec);
byte[] encryptedBytes = Base64.getDecoder().decode(encryptedText);
byte[] decryptedBytes = cipher.doFinal(encryptedBytes);
return new String(decryptedBytes);
}
}
```
其中,`encrypt`方法接受两个参数:待加密的明文和密钥,返回加密后的密文;`decrypt`方法接受两个参数:待解密的密文和密钥,返回解密后的明文。这里使用的是AES算法,采用ECB模式和PKCS5Padding填充方式。在实际应用中,需要注意密钥的安全性和密文的传输安全性。
阅读全文
相关推荐
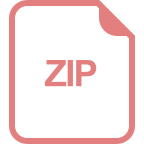
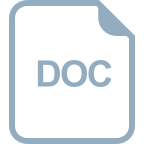
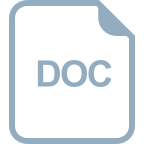
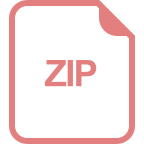
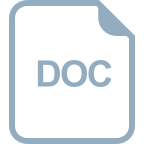
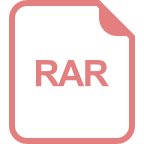
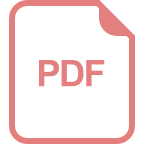
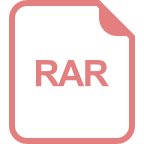
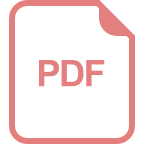
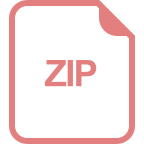
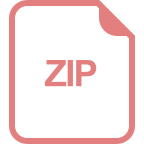
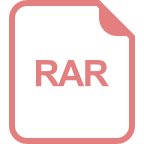
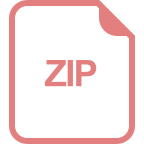
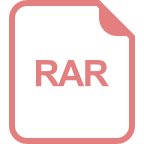
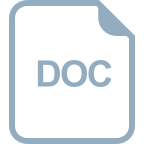
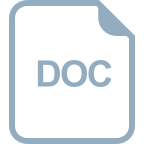
