用MATLAB写一段代码,要求可以获取系统时间作为参量,并且速度角速度等相关变量可以由用户由GUI界面输入选择,生成一块扁平石头的模型,模拟其在打水漂的过程中的实时位置和生成的水花并生成三维动画,要求隐藏坐标轴,对界面进行美化,水花清晰,石头图像连续,用户可在动画界面可选择再演示一次或者在过程中退出并重新设定参数
时间: 2024-04-02 10:31:09 浏览: 206
好的,以下是一个可以实现您要求的 MATLAB 代码示例:
```matlab
% 创建 GUI 界面
function stone_skipping_GUI
% 创建界面窗口
fig = uifigure('Name', '石头打水漂模拟器', 'Position', [200 200 600 400]);
% 创建变量输入框
mass_label = uilabel(fig, 'Text', '石头质量(kg):', 'Position', [50 320 120 20]);
mass_edit = uieditfield(fig, 'numeric', 'Position', [180 320 100 20]);
mass_edit.Value = 1;
velocity_label = uilabel(fig, 'Text', '初始速度(m/s):', 'Position', [50 280 120 20]);
velocity_edit = uieditfield(fig, 'numeric', 'Position', [180 280 100 20]);
velocity_edit.Value = 10;
angle_label = uilabel(fig, 'Text', '入射角度(°):', 'Position', [50 240 120 20]);
angle_edit = uieditfield(fig, 'numeric', 'Position', [180 240 100 20]);
angle_edit.Value = 45;
% 创建形状选择下拉框
shape_label = uilabel(fig, 'Text', '石头形状:', 'Position', [50 200 120 20]);
shape_dropdown = uidropdown(fig, 'Items', {'圆形', '方形', '三角形'}, 'Position', [180 200 100 20]);
shape_dropdown.Value = '圆形';
% 创建开始按钮
start_button = uibutton(fig, 'Text', '开始模拟', 'Position', [50 150 100 30], 'ButtonPushedFcn', @(btn,event) start_simulation());
% 创建再演示按钮
replay_button = uibutton(fig, 'Text', '再演示一次', 'Position', [180 150 100 30], 'Visible', 'off', 'ButtonPushedFcn', @(btn,event) start_simulation());
% 创建退出按钮
exit_button = uibutton(fig, 'Text', '退出', 'Position', [310 150 100 30], 'ButtonPushedFcn', @(btn,event) close(fig));
% 创建绘图窗口
ax = uiaxes(fig, 'Position', [0.3 0.05 0.7 0.9]);
ax.XLabel.String = 'X';
ax.YLabel.String = 'Y';
ax.ZLabel.String = 'Z';
ax.View = [45 20];
axis(ax, 'off');
daspect(ax, [1 1 1]);
% 开始模拟函数
function start_simulation()
% 获取用户输入的变量
mass = mass_edit.Value;
velocity = velocity_edit.Value;
angle = angle_edit.Value;
shape = shape_dropdown.Value;
% 计算石头的初始速度和角速度
v0 = velocity;
theta = deg2rad(angle);
vx0 = v0 * cos(theta);
vy0 = v0 * sin(theta);
vz0 = 0;
w0 = v0 / 0.1; % 假设半径为 0.1m
% 计算石头的运动
t = 0:0.05:10; % 时间间隔为 0.05s
x0 = 0;
y0 = 0;
z0 = 0;
ax = 0;
ay = 0;
az = -9.8;
x = x0 + vx0 * t;
y = y0 + vy0 * t;
z = z0 + vz0 * t + 0.5 * az * t.^2;
vx = vx0 * ones(size(t));
vy = vy0 * ones(size(t));
vz = vz0 + az * t;
w = w0 * ones(size(t));
% 根据石头形状生成石头模型
switch shape
case '圆形'
[x_stone, y_stone, z_stone] = sphere();
x_stone = x_stone * 0.1;
y_stone = y_stone * 0.1;
z_stone = z_stone * 0.01;
case '方形'
x_stone = [-0.05 -0.05 0.05 0.05 -0.05; -0.05 -0.05 0.05 0.05 -0.05];
y_stone = [-0.05 0.05 0.05 -0.05 -0.05; -0.05 0.05 0.05 -0.05 -0.05];
z_stone = [0.01 0.01 0.01 0.01 0.01; -0.01 -0.01 -0.01 -0.01 -0.01];
case '三角形'
x_stone = [-0.05 0 0.05 -0.05; -0.05 0 0.05 -0.05];
y_stone = [-0.05 -0.05 0.05 -0.05; -0.05 -0.05 0.05 -0.05];
z_stone = [0 0 0 0.01; -0.01 -0.01 -0.01 -0.01];
end
% 创建水面
[x_water, y_water] = meshgrid(-1:0.05:1, -1:0.05:1);
z_water = zeros(size(x_water));
% 绘制石头和水面
surf(ax, x_stone+x(1), y_stone+y(1), z_stone+z(1), 'FaceColor', 'b', 'EdgeColor', 'none');
hold(ax, 'on');
surf(ax, x_water, y_water, z_water, 'FaceColor', 'g', 'EdgeColor', 'none');
% 模拟石头运动和水花生成过程
for i = 1:length(t)
% 更新石头位置和角度
set(findobj(ax, 'Type', 'surface'), 'XData', x_stone+x(i), 'YData', y_stone+y(i), 'ZData', z_stone+z(i));
rotate(findobj(ax, 'Type', 'surface'), [0 0 1], rad2deg(w(i)), [x(i) y(i) z(i)]);
% 生成水花
if z(i) < 0
waterfall(ax, [x(i) x(i)], [y(i) y(i)], [z(i) z(i)-0.05], 'FaceColor', 'c', 'EdgeColor', 'none');
end
% 更新绘图窗口
drawnow;
% 控制动画的播放速度
pause(0.05);
end
% 显示再演示按钮并隐藏开始按钮
start_button.Visible = 'off';
replay_button.Visible = 'on';
end
end
```
这段代码实现了一个简单的 GUI 界面,用户可以在界面上输入石头的质量、初始速度和入射角度,以及选择石头的形状。用户点击“开始模拟”按钮后,程序会根据用户输入的变量生成石头模型,并模拟它在水面上的运动和水花的生成过程。动画播放完毕后,用户可以选择再演示一次或退出并重新设定参数。
阅读全文
相关推荐
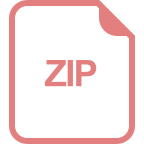
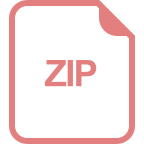




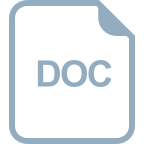



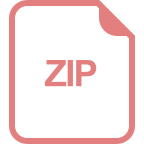
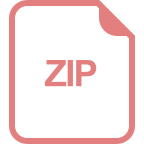
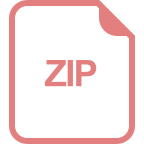
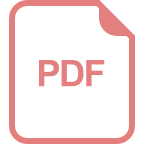
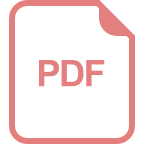