java实现RabbitMQ连接池
时间: 2023-07-06 08:40:26 浏览: 60
以下是一个简单的Java实现RabbitMQ连接池的示例代码:
1. 首先,定义一个RabbitMQ连接池类,该类使用Apache Commons Pool实现连接池功能。
```java
public class RabbitMQPool {
private static final Logger LOGGER = LoggerFactory.getLogger(RabbitMQPool.class);
private final GenericObjectPool<Connection> pool;
public RabbitMQPool(ConnectionFactory connectionFactory) {
pool = new GenericObjectPool<>(new ConnectionFactoryPoolableObjectFactory(connectionFactory));
pool.setMaxIdle(10);
pool.setMaxTotal(100);
pool.setMinIdle(5);
pool.setTestOnBorrow(true);
}
public Connection getConnection() throws Exception {
LOGGER.debug("Get RabbitMQ Connection from Pool");
return pool.borrowObject();
}
public void releaseConnection(Connection connection) {
LOGGER.debug("Release RabbitMQ Connection to Pool");
pool.returnObject(connection);
}
public void close() {
LOGGER.debug("Close RabbitMQ Connection Pool");
pool.close();
}
private static class ConnectionFactoryPoolableObjectFactory extends BasePoolableObjectFactory<Connection> {
private final ConnectionFactory connectionFactory;
public ConnectionFactoryPoolableObjectFactory(ConnectionFactory connectionFactory) {
this.connectionFactory = connectionFactory;
}
@Override
public Connection makeObject() throws Exception {
LOGGER.debug("Create RabbitMQ Connection");
return connectionFactory.newConnection();
}
@Override
public void destroyObject(Connection connection) throws Exception {
LOGGER.debug("Destroy RabbitMQ Connection");
connection.close();
}
@Override
public boolean validateObject(Connection connection) {
LOGGER.debug("Validate RabbitMQ Connection");
return connection.isOpen();
}
}
}
```
2. 在应用程序中使用RabbitMQ连接池获取连接并进行消息传递。
```java
public class RabbitMQClient {
private static final Logger LOGGER = LoggerFactory.getLogger(RabbitMQClient.class);
private final RabbitMQPool rabbitMQPool;
public RabbitMQClient(ConnectionFactory connectionFactory) {
rabbitMQPool = new RabbitMQPool(connectionFactory);
}
public void sendMessage(String message, String queueName) {
try (Connection connection = rabbitMQPool.getConnection();
Channel channel = connection.createChannel()) {
channel.queueDeclare(queueName, false, false, false, null);
channel.basicPublish("", queueName, null, message.getBytes());
LOGGER.debug("Sent message '{}' to queue '{}'", message, queueName);
} catch (Exception e) {
LOGGER.error("Failed to send message '{}' to queue '{}'", message, queueName, e);
}
}
public void close() {
rabbitMQPool.close();
}
}
```
在上述示例代码中,RabbitMQPool类是一个连接池实现,用于管理RabbitMQ连接。ConnectionFactoryPoolableObjectFactory类是一个ObjectPoolableObjectFactory实现,用于创建和销毁RabbitMQ连接。RabbitMQClient类是一个使用RabbitMQ连接池的客户端实现,用于发送消息到RabbitMQ消息队列。
相关推荐
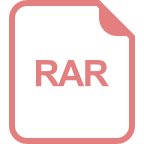
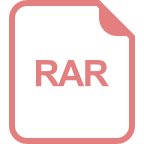
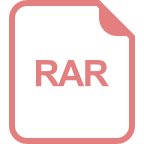












