c++实现扫雷内部数组生成,要求10x26的格式,用“”号表示雷,非雷位置用0-8表示,共有50颗雷。
时间: 2024-05-16 16:13:26 浏览: 100
以下是一个简单的 C++ 实现,生成一个 10x26 的扫雷内部数组,其中有 50 颗雷,用双引号表示雷,非雷位置用 0-8 表示:
```c++
#include <iostream>
#include <random>
#include <algorithm>
#include <vector>
int main() {
const int ROWS = 10;
const int COLS = 26;
const int NUM_MINES = 50;
// Initialize the board with all zeros
int board[ROWS][COLS] = {0};
// Generate a vector of all positions on the board
std::vector<std::pair<int, int>> positions;
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
positions.push_back(std::make_pair(i, j));
}
}
// Shuffle the positions vector
std::random_device rd;
std::mt19937 g(rd());
std::shuffle(positions.begin(), positions.end(), g);
// Place mines on the board
for (int i = 0; i < NUM_MINES; i++) {
int row = positions[i].first;
int col = positions[i].second;
board[row][col] = -1;
}
// Fill in the numbers around the mines
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == -1) {
for (int k = -1; k <= 1; k++) {
for (int l = -1; l <= 1; l++) {
int r = i + k;
int c = j + l;
if (r >= 0 && r < ROWS && c >= 0 && c < COLS && board[r][c] != -1) {
board[r][c]++;
}
}
}
}
}
}
// Print out the board
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] == -1) {
std::cout << "\"\" ";
} else {
std::cout << board[i][j] << " ";
}
}
std::cout << std::endl;
}
return 0;
}
```
这个程序使用了 C++ 的随机数生成器和 STL 容器,将所有位置随机打乱,然后在前 50 个位置上放置雷。接下来,遍历所有位置,在雷周围的位置上标记数字。最后打印出扫雷内部数组,用双引号表示雷,非雷位置用 0-8 表示。
阅读全文
相关推荐
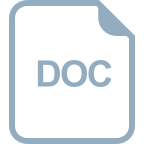
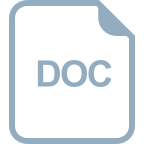
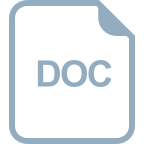

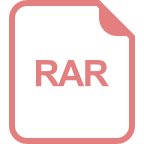
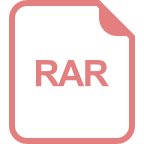
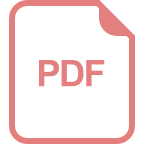
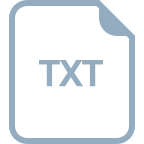
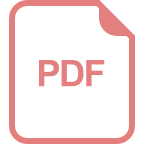
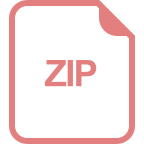







