python实现网上购物系统
时间: 2023-11-04 12:05:05 浏览: 47
要实现网上购物系统,需要考虑以下几个方面:
1. 商品管理:实现商品的添加、修改、删除、查询等功能。
2. 用户管理:实现用户的注册、登录、修改个人信息、查看购物记录等功能。
3. 购物车管理:实现用户将商品加入购物车、修改购物车中商品数量、删除购物车中商品等功能。
4. 订单管理:实现用户提交订单、查看订单详情、取消订单等功能。
5. 支付管理:实现用户在下单后选择支付方式,完成支付流程等功能。
下面是一个简单的示例代码:
```python
class Product:
def __init__(self, name, price, description):
self.name = name
self.price = price
self.description = description
class ShoppingCart:
def __init__(self):
self.items = {}
def add_item(self, product, quantity):
if product in self.items:
self.items[product] += quantity
else:
self.items[product] = quantity
def remove_item(self, product, quantity):
if product in self.items:
if quantity >= self.items[product]:
del self.items[product]
else:
self.items[product] -= quantity
def get_total(self):
return sum([product.price * self.items[product] for product in self.items])
class Order:
def __init__(self, user, cart, payment_method):
self.user = user
self.cart = cart
self.payment_method = payment_method
def place_order(self):
print(f"Placing order for {self.user} using {self.payment_method}")
print("Order details:")
for product in self.cart.items:
print(f"{product.name} ({self.cart.items[product]} units) - ${product.price * self.cart.items[product]}")
print(f"Total cost: ${self.cart.get_total()}")
class User:
def __init__(self, username, password):
self.username = username
self.password = password
self.shopping_cart = ShoppingCart()
self.orders = []
def add_to_cart(self, product, quantity):
self.shopping_cart.add_item(product, quantity)
def remove_from_cart(self, product, quantity):
self.shopping_cart.remove_item(product, quantity)
def place_order(self, payment_method):
order = Order(self, self.shopping_cart, payment_method)
order.place_order()
self.orders.append(order)
# example usage
apple = Product("Apple", 1.0, "A fresh apple")
banana = Product("Banana", 1.5, "A ripe banana")
user = User("test", "password")
user.add_to_cart(apple, 2)
user.add_to_cart(banana, 3)
user.place_order("Credit card")
```
当然,这只是一个简单的实现示例,实际的网上购物系统需要更多的功能和复杂的实现,例如商品分类、搜索、评价、物流、退换货等等。
阅读全文
相关推荐
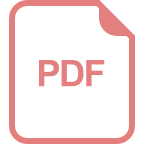
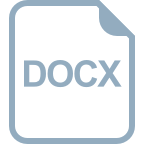
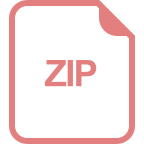
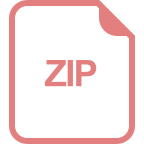
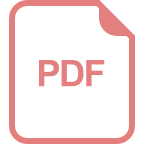
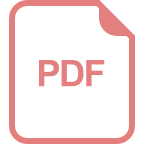
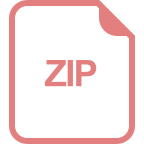
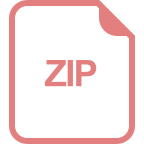
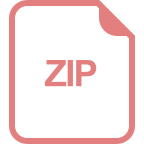
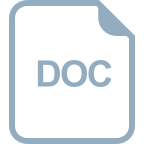
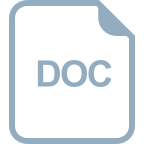
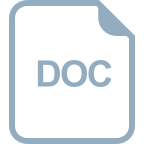
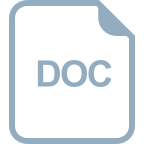
