java将文件资源下载至本地
时间: 2024-03-11 16:50:40 浏览: 25
以下是一个简单的Java实现将文件资源下载至本地的示例代码:
```java
public void downloadFile(String url, String filePath) throws IOException {
URL fileUrl = new URL(url);
HttpURLConnection connection = (HttpURLConnection) fileUrl.openConnection();
connection.setRequestMethod("GET");
// 获取文件大小
int contentLength = connection.getContentLength();
// 创建文件输出流
FileOutputStream outputStream = new FileOutputStream(filePath);
// 读取文件流并写入文件
InputStream inputStream = connection.getInputStream();
byte[] buffer = new byte[4096];
int bytesRead = -1;
int totalBytesRead = 0;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
totalBytesRead += bytesRead;
System.out.println(String.format("Downloaded %d/%d bytes", totalBytesRead, contentLength));
}
// 关闭流
outputStream.close();
inputStream.close();
}
```
在上面的代码中,我们通过 `URL` 类来创建一个文件资源的 URL 对象,并打开一个 `HttpURLConnection` 连接。然后,我们设置请求方法为 GET,并获取文件大小。接着,我们创建一个文件输出流,并通过 `InputStream` 类获取文件流。在读取文件流时,我们将每次读取的数据写入文件,并输出下载进度。最后,我们关闭流并结束下载。
你需要传入两个参数:文件资源的 URL 和本地文件保存的路径。例如,如果你要下载一个名为 `example.zip` 的文件,可以调用以下方法:
```java
downloadFile("http://example.com/example.zip", "C:/Downloads/example.zip");
```
这将把文件下载到本地的 `C:/Downloads` 目录下。
相关推荐
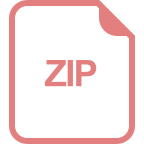














