c# json文件读写
时间: 2023-08-29 15:11:39 浏览: 50
在 C# 中,你可以使用 `System.Text.Json` 命名空间来读写 JSON 文件。下面是一个简单的示例:
```csharp
using System;
using System.IO;
using System.Text.Json;
namespace JsonReadWriteExample
{
class Program
{
static void Main(string[] args)
{
// 读取 JSON 文件
string jsonText = File.ReadAllText("data.json");
// 解析 JSON 数据
var jsonObject = JsonDocument.Parse(jsonText);
// 读取属性值
string name = jsonObject.RootElement.GetProperty("name").GetString();
int age = jsonObject.RootElement.GetProperty("age").GetInt32();
Console.WriteLine($"Name: {name}");
Console.WriteLine($"Age: {age}");
// 修改属性值
var newJsonObject = new JsonObject
{
{ "name", "John" },
{ "age", 30 }
};
// 将修改后的 JSON 数据写入文件
File.WriteAllText("data.json", newJsonObject.ToString());
}
}
}
```
在上面的示例中,我们首先使用 `File.ReadAllText` 方法将 JSON 文件的内容读取到一个字符串中。然后,使用 `JsonDocument.Parse` 方法解析 JSON 数据,并使用 `GetProperty` 方法读取属性的值。
如果你需要修改 JSON 数据,你可以创建一个新的 `JsonObject` 对象,设置新的属性值,然后使用 `ToString` 方法将其转换为字符串,并使用 `File.WriteAllText` 方法将其写入文件。
请确保在运行示例代码之前,将 `data.json` 替换为你实际的 JSON 文件路径。
相关推荐












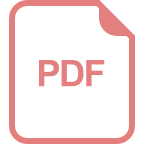
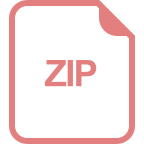