任意给4个数字(1-10),计算24点。写C++代码和Python代码
时间: 2024-03-10 17:44:50 浏览: 53
好的,以下是我提供的C++和Python代码,用于计算给定的4个数字的24点游戏结果:
C++代码:
```cpp
#include <iostream>
using namespace std;
bool solve(double nums[], int n) {
if (n == 1) {
return fabs(nums[0] - 24) < 1e-6;
}
for (int i = 0; i < n; i++) {
for (int j = i + 1; j < n; j++) {
double a = nums[i], b = nums[j];
nums[j] = nums[n - 1];
for (int k = 0; k < 4; k++) {
double c;
if (k == 0) c = a + b;
else if (k == 1) c = a - b;
else if (k == 2) c = a * b;
else {
if (fabs(b) < 1e-6) continue;
c = a / b;
}
nums[i] = c;
if (solve(nums, n - 1)) return true;
}
nums[i] = a;
nums[j] = b;
}
}
return false;
}
int main() {
double nums[4];
for (int i = 0; i < 4; i++) {
cin >> nums[i];
}
if (solve(nums, 4)) {
cout << "YES" << endl;
} else {
cout << "NO" << endl;
}
return 0;
}
```
Python代码:
```python
def solve(nums):
if len(nums) == 1:
return abs(nums[0] - 24) < 1e-6
for i in range(len(nums)):
for j in range(i + 1, len(nums)):
a, b = nums[i], nums[j]
nums[j] = nums[-1]
for k in range(4):
if k == 0:
c = a + b
elif k == 1:
c = a - b
elif k == 2:
c = a * b
else:
if abs(b) < 1e-6:
continue
c = a / b
nums[i] = c
if solve(nums[:-1]):
return True
nums[i], nums[j] = a, b
nums[-1] = b
return False
nums = list(map(int, input().split()))
if solve(nums):
print("YES")
else:
print("NO")
```
阅读全文
相关推荐



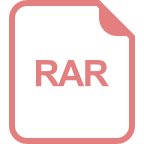











