用python编写一段读写文件的代码
时间: 2024-06-02 11:07:22 浏览: 107
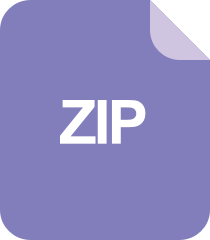
python-etc:较小的Python示例和功能代码段
当然,我可以为您提供这样的代码:
# 写文件
with open('file.txt', 'w') as f:
f.write('Hello World!')
# 读文件
with open('file.txt', 'r') as f:
content = f.read()
print(content)
这段代码会在当前目录下创建一个名为 file.txt 的文件,并写入字符串 "Hello World!"。然后,它会再次打开文件并读取其中的内容,并将内容输出到控制台上。该代码使用 with 关键字来确保安全地打开和关闭文件,从而避免了潜在的资源泄漏问题。
阅读全文
相关推荐
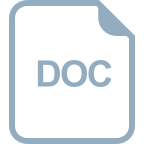
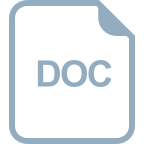



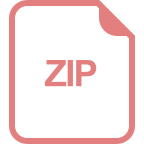
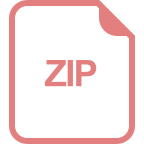
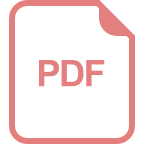
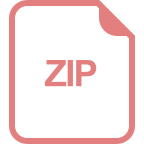
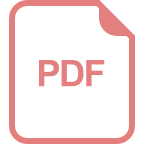
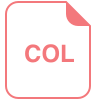
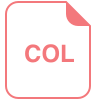





