编制生产者—消费者算法,模拟 1 个生产者、3 个消费者进行同步的操作
时间: 2023-04-11 12:00:32 浏览: 163
好的,我可以回答这个问题。生产者-消费者问题是一个经典的同步问题,可以使用信号量来实现。具体实现方式可以使用一个缓冲区来存储生产者生产的数据,当缓冲区满时,生产者需要等待,直到有消费者消费数据,缓冲区有空位时,生产者才能继续生产。同样,当缓冲区为空时,消费者需要等待,直到有生产者生产数据,缓冲区有数据时,消费者才能继续消费。在这个问题中,可以使用一个互斥锁来保证同时只有一个线程访问缓冲区,避免竞争条件的发生。
相关问题
编程实现生产者—消费者算法,模拟 1 个生产者、3 个消费者进行同步的操作
好的,这个问题我可以回答。生产者-消费者问题是一个经典的同步问题,可以使用多种方法来实现。其中一种常见的方法是使用信号量来进行同步操作。具体实现可以使用一个计数器来表示缓冲区中可用的空间数量,当生产者生产一个数据时,计数器减一;当消费者消费一个数据时,计数器加一。当计数器为0时,消费者需要等待生产者生产数据;当计数器为缓冲区大小时,生产者需要等待消费者消费数据。这样可以保证生产者和消费者之间的同步操作。
具体实现可以参考以下代码:
```python
from threading import Thread, Semaphore
import time
BUFFER_SIZE = 5 # 缓冲区大小
empty = Semaphore(BUFFER_SIZE) # 空闲空间数量
full = Semaphore(0) # 已使用空间数量
mutex = Semaphore(1) # 互斥锁
buffer = [None] * BUFFER_SIZE # 缓冲区
def producer():
global buffer
for i in range(10):
empty.acquire() # 等待空闲空间
mutex.acquire() # 获取互斥锁
buffer[i % BUFFER_SIZE] = i # 生产数据
print(f"生产者生产了数据 {i}")
mutex.release() # 释放互斥锁
full.release() # 增加已使用空间数量
time.sleep(1)
def consumer(id):
global buffer
while True:
full.acquire() # 等待已使用空间
mutex.acquire() # 获取互斥锁
data = buffer.pop(0) # 消费数据
print(f"消费者{id}消费了数据 {data}")
mutex.release() # 释放互斥锁
empty.release() # 增加空闲空间数量
time.sleep(1)
if __name__ == "__main__":
t_producer = Thread(target=producer)
t_consumer1 = Thread(target=consumer, args=(1,))
t_consumer2 = Thread(target=consumer, args=(2,))
t_consumer3 = Thread(target=consumer, args=(3,))
t_producer.start()
t_consumer1.start()
t_consumer2.start()
t_consumer3.start()
t_producer.join()
t_consumer1.join()
t_consumer2.join()
t_consumer3.join()
```
这个代码实现了一个生产者和三个消费者之间的同步操作,生产者每隔一秒钟生产一个数据,消费者每隔一秒钟消费一个数据。当缓冲区已满时,生产者需要等待;当缓冲区为空时,消费者需要等待。这样可以保证生产者和消费者之间的同步操作,避免了数据竞争和死锁问题。
生产者-消费者算法模拟
生产者-消费者算法是一种经典的进程同步算法,用于解决生产者和消费者之间的同步和互斥问题。在该算法中,生产者进程负责向缓冲池中生产产品,而消费者进程则负责从缓冲池中消费产品。为了保证生产者和消费者之间的同步和互斥,需要使用互斥锁和条件变量来实现。
在C++中,可以通过定义生产者和消费者类来实现生产者-消费者算法的模拟。在这些类中,可以定义一个缓冲池,用于存储生产者生产的产品,并且使用互斥锁来保证对缓冲池的互斥访问。同时,还需要定义一个条件变量,用于在缓冲池为空或者已满时进行等待和唤醒操作。
在生产者类中,可以定义一个生产函数,用于向缓冲池中添加产品。在该函数中,需要先获取互斥锁,然后判断缓冲池是否已满,如果已满则等待条件变量,否则向缓冲池中添加产品,并且唤醒等待在条件变量上的消费者进程。最后,释放互斥锁。
在消费者类中,可以定义一个消费函数,用于从缓冲池中消费产品。在该函数中,需要先获取互斥锁,然后判断缓冲池是否为空,如果为空则等待条件变量,否则从缓冲池中取出产品,并且唤醒等待在条件变量上的生产者进程。最后,释放互斥锁。
在主函数中,可以创建一个生产者对象和一个消费者对象,并且循环调用它们的生产和消费函数,以模拟生产者-消费者算法的运行过程。每次调用后,可以输出缓冲池的产品状态和数量,以便观察算法的运行情况。
相关推荐
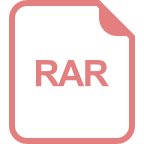
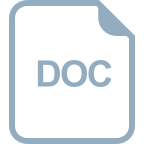












