在hnwl.jdxy包中定义一个Shape接口,该接口中包含成员属性PI=3.1415926、计算面积的方法getArea( )和计算周长的方法getPerimeter( ),在hnwl.jdxy包中设计一个实现Shape接口的圆类Circle, 类Circle包含成员属性:圆心坐标x、y及圆半径r,以及设置及获取各属性的成员方法。再在包hnwl.gjxy中定义一个圆柱体类Cylinder, Cylinder 继承自Circle类,增加了属性:高度h,增加了设置和获取h的成员方法、以及计算表面积的方法getArea( )和计算体积的方法getVolume( )。最后在hnwl.a.b包下创建测试类Test ,在Test类的main( )中创建一个Circle类的对象,显示其所有属性,计算并显示其面积和周长,再创建一个Cylinder类的对象,显示其所有属性,计算并显示其表面积和体积。
时间: 2024-02-15 08:01:30 浏览: 166
好的,以下是你所要求的Java程序:
在hnwl.jdxy包中定义Shape接口:
```java
package hnwl.jdxy;
public interface Shape {
double PI = 3.1415926;
double getArea();
double getPerimeter();
}
```
在hnwl.jdxy包中定义实现Shape接口的圆类Circle:
```java
package hnwl.jdxy;
public class Circle implements Shape {
private double x;
private double y;
private double r;
public Circle(double x, double y, double r) {
this.x = x;
this.y = y;
this.r = r;
}
public void setX(double x) {
this.x = x;
}
public double getX() {
return x;
}
public void setY(double y) {
this.y = y;
}
public double getY() {
return y;
}
public void setR(double r) {
this.r = r;
}
public double getR() {
return r;
}
@Override
public double getArea() {
return PI * r * r;
}
@Override
public double getPerimeter() {
return 2 * PI * r;
}
}
```
在hnwl.gjxy包中定义圆柱体类Cylinder,继承自Circle:
```java
package hnwl.gjxy;
import hnwl.jdxy.Shape;
import hnwl.jdxy.Circle;
public class Cylinder extends Circle {
private double h;
public Cylinder(double x, double y, double r, double h) {
super(x, y, r);
this.h = h;
}
public void setH(double h) {
this.h = h;
}
public double getH() {
return h;
}
public double getVolume() {
return getArea() * h;
}
@Override
public double getArea() {
return 2 * super.getArea() + super.getPerimeter() * h;
}
}
```
在hnwl.a.b包下创建测试类Test:
```java
package hnwl.a.b;
import hnwl.gjxy.Cylinder;
import hnwl.jdxy.Circle;
public class Test {
public static void main(String[] args) {
Circle circle = new Circle(0, 0, 5);
System.out.println("圆的属性:");
System.out.println("圆心坐标:(" + circle.getX() + ", " + circle.getY() + ")");
System.out.println("半径:" + circle.getR());
System.out.println("面积:" + circle.getArea());
System.out.println("周长:" + circle.getPerimeter());
Cylinder cylinder = new Cylinder(0, 0, 5, 10);
System.out.println("圆柱体的属性:");
System.out.println("圆心坐标:(" + cylinder.getX() + ", " + cylinder.getY() + ")");
System.out.println("半径:" + cylinder.getR());
System.out.println("高度:" + cylinder.getH());
System.out.println("表面积:" + cylinder.getArea());
System.out.println("体积:" + cylinder.getVolume());
}
}
```
注意:以上程序中的所有类都必须放在对应的包中,否则会编译错误。
阅读全文
相关推荐


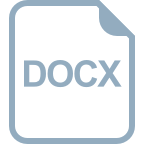
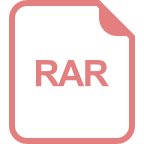
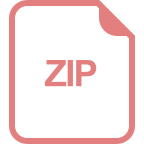
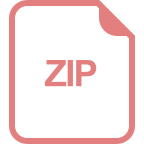
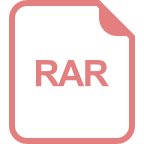
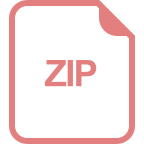
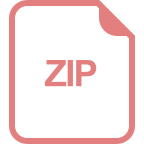
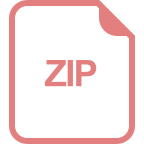