如何设计和实现一个C++类Line来表示直线,其中包括一个用于计算两条直线交点的友元函数?并且,请提供一个详细的步骤或示例,在主函数main()中创建并测试这个Line类的对象。
时间: 2024-11-27 13:21:55 浏览: 4
设计一个C++类`Line`表示直线,首先需要包含直线的基本属性,如两个端点(x1, y1)和(x2, y2)。我们还需要实现计算两条直线交点的方法,可以利用线性方程组解法或者向量叉积等数学原理。
```cpp
#include <iostream>
#include <cmath>
// 定义Point类作为Line的组成部分
class Point {
public:
double x, y;
Point(double x = 0, double y = 0): x(x), y(y) {}
};
// Line类,包括两条直线的端点
class Line {
private:
Point p1, p2;
public:
// 构造函数
Line(Point p1, Point p2): p1(p1), p2(p2) {}
// 友元函数,计算两条线的交点
friend Point intersection(const Line& line1, const Line& line2);
// 省略其他必要的操作,如打印、比较等...
};
// 计算两条线的交点,假设这两条线都是非平行的
Point intersection(const Line& line1, const Line& line2) {
double slope1 = (line2.p2.y - line1.p2.y) / (line2.p2.x - line1.p2.x);
double slope2 = (line1.p1.y - line2.p1.y) / (line1.p1.x - line2.p1.x);
if (slope1 == slope2) {
// 平行情况,不存在交点
return Point();
} else {
double y_intercept1 = line1.p1.y - slope1 * line1.p1.x;
double y_intercept2 = line2.p1.y - slope2 * line2.p1.x;
return Point(slope1 * (y_intercept2 - y_intercept1) + line1.p1.x, y_intercept1);
}
}
int main() {
// 创建两条直线实例
Line line1(Point(1, 2), Point(4, 6));
Line line2(Point(-1, 3), Point(3, 5));
// 计算交点
Point intersectionPoint = intersection(line1, line2);
// 如果有交点,打印结果
if (!intersectionPoint.isOrigin()) { // 判断是否为原点
std::cout << "The intersection point is (" << intersectionPoint.x << ", " << intersectionPoint.y << ").\n";
} else {
std::cout << "Lines are parallel or identical.\n";
}
return 0;
}
```
在这个例子中,`Line`类有两个端点,`intersection()`函数是它的友元函数,用来计算两条线的交点。在`main()`函数中,我们创建了两条线并调用了`intersection()`函数。如果交点不是原点,就显示出来;否则,说明线是平行的。
阅读全文
相关推荐
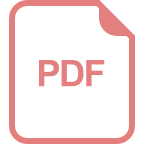
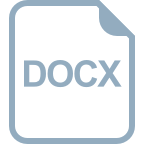
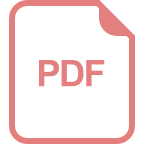
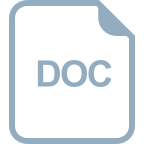





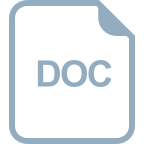
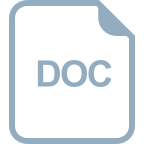
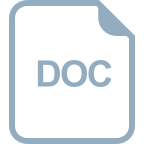
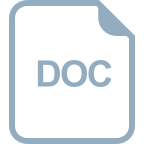
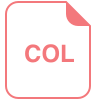


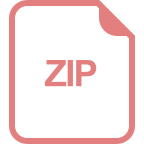
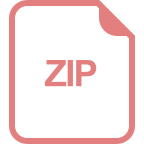