WebPImageReader 使用这个需要引入什么依赖Java
时间: 2024-11-05 21:34:26 浏览: 12
WebPImageReader 是一个用于处理 WebP 图像格式的 Java 类,它通常与 Android 平台中的库相关,特别是那些支持图像解码功能的部分。如果你要在 Android 中使用 WebPImageReader,你需要引入 Android 开发工具包 (Android SDK) 中的 ExifInterface 和 BitmapFactory 类,这两个库提供了解析图像元数据和加载位图的功能。
具体的依赖项通常是在 Gradle 构建脚本中添加的,对于 Android Studio 或者 Kotlin 项目,你会看到类似这样的代码片段:
```groovy
dependencies {
implementation 'com.android.support:exifinterface:...'
implementation 'androidx.appcompat:appcompat:...'
}
```
这里的 `com.android.support:exifinterface` 引入了 ExifInterface 类,而 `androidx.appcompat:appcompat` 包含了 BitmapFactory 的实现。请确保更新版本号到最新的兼容库。
如果你在纯 Java 项目中使用,可能会需要额外的第三方库,如 OpenCV 或其他图像处理库,它们可能也包含对 WebP 的支持。
相关问题
WebPImageReader 使用这个需要引入什么依赖Java的pom
WebPImageReader通常用于处理WebP图像格式,要在Java项目中使用它,你需要添加Google的插件库gson和webp的相关依赖。如果你使用Maven来管理项目依赖,可以在`pom.xml`文件中添加以下内容:
```xml
<dependencies>
<!-- Google Gson for JSON support -->
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.6</version> <!-- 或者最新的稳定版本 -->
</dependency>
<!-- For decoding WebP images -->
<dependency>
<groupId>com.github.bumptech.glide</groupId>
<artifactId>glide</artifactId>
<version>4.12.0</version> <!-- 或者最新的Glide版本,包括对WebP的支持 -->
<exclusions>
<!-- Exclude the old WebP library to use Google's newer one -->
<exclusion>
<groupId>com.android.support</groupId>
<artifactId>support-compat</artifactId>
</exclusion>
<exclusion>
<groupId>com.android.support</groupId>
<artifactId>support-media-compat</artifactId>
</exclusion>
<exclusion>
<groupId>com.android.support</groupId>
<artifactId>support-v4</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>com.google.android.webp</groupId>
<artifactId>webpdecoder</artifactId>
<version>1.1.0-rc0</version> <!-- 最新的WebP解码器版本 -->
</dependency>
</dependencies>
```
确保替换掉版本号为你项目所需的最新版本。然后运行`mvn install`命令来下载并整合这些依赖。
Java读取图片分辨率直接webp文件
在Java中,直接读取WebP图片的分辨率需要借助一些库来支持WebP格式。WebP是由Google开发的一种高效的图像格式,如果你想要处理这种格式,可以使用`java-jai-imageio`库,它包含了对WebP的支持。
首先,你需要在项目中添加WebP相关的依赖,例如Maven中:
```xml
<dependency>
<groupId>com.sun.media.jai</groupId>
<artifactId>jai_imageio</artifactId>
<version>1.1.4</version>
</dependency>
```
然后,你可以使用以下代码片段来获取WebP图片的宽度和高度:
```java
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
public class WebPImageReader {
public static void main(String[] args) throws Exception {
String webpFilePath = "path_to_your_webp_file.webp";
BufferedImage image = ImageIO.read(webpFilePath);
int width = image.getWidth();
int height = image.getHeight();
System.out.println("Image resolution: " + width + "x" + height);
}
}
```
这段代码会打开指定路径的WebP文件,并将其转换成`BufferedImage`对象,从这个对象可以直接获取到宽度和高度信息。
阅读全文
相关推荐
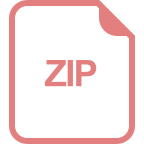
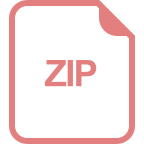
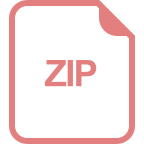
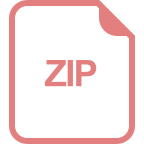
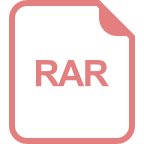
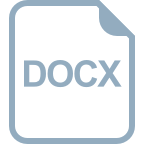
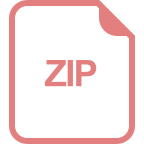
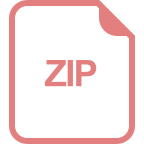
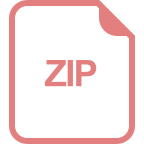
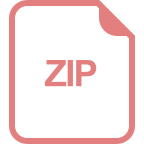
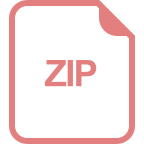
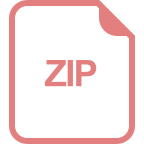
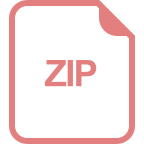
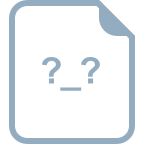