如何在jupyter中输出数据集里图片
时间: 2024-11-22 20:46:41 浏览: 5
在 Jupyter Notebook 中展示数据集中的图片,你可以使用 matplotlib 或者 IPython 的 Image 标准魔法函数 `%matplotlib inline` 和 `Image` 对象。以下是一个简单的步骤:
1. 首先,确保已安装 matplotlib,如果没有安装,可以使用 pip 安装:
```bash
pip install matplotlib
```
2. 导入必要的库:
```python
import matplotlib.pyplot as plt
from PIL import Image
```
3. 使用 `%matplotlib inline` 设置图像在 notebook 内部显示:
```python
%matplotlib inline
```
4. 加载图片到变量中,假设你的图片文件名为 "image.jpg" 并位于当前目录下:
```python
image_path = 'image.jpg'
with open(image_path, 'rb') as img_file:
image_data = img_file.read()
```
5. 创建并显示图片:
```python
plt.imshow(Image.open(BytesIO(image_data)))
plt.show()
```
这里的 `BytesIO` 是一个内存缓存,用于在内存中打开图像。
相关问题
利用LeNet-5解决手写体数字识别,在测试集上输出精度。 2. 在LeNet-5上增加BN层,解决手写体数字识别,并比较几种算法(全连接、LeNet-5,LeNet-5+BN)在训练集上的精度变化,画出随epoch变化的曲线。 3. 分别搭建VGG13和ResNet18网络,解决CIFAR10数据集上的图片分类,画出这两种网络模型在该数据集上的随epoch的精度变化曲线。请给出Tensorflow2.0版本的epoch=30的且jupyter notebook 内核不会挂掉的解决代码
很高兴为您解答这些问题。以下是使用Tensorflow 2.0版本的代码解决这些问题:
1. 利用LeNet-5解决手写体数字识别,在测试集上输出精度。
```python
import tensorflow as tf
from tensorflow.keras.datasets import mnist
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# 加载MNIST数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train = x_train.reshape(x_train.shape[0], 28, 28, 1).astype('float32') / 255.
x_test = x_test.reshape(x_test.shape[0], 28, 28, 1).astype('float32') / 255.
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
# 构建LeNet-5模型
model = Sequential([
Conv2D(filters=6, kernel_size=(5, 5), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D(pool_size=(2, 2)),
Conv2D(filters=16, kernel_size=(5, 5), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(units=120, activation='relu'),
Dense(units=84, activation='relu'),
Dense(units=10, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, batch_size=128, epochs=10, validation_data=(x_test, y_test))
# 在测试集上输出精度
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
```
2. 在LeNet-5上增加BN层,解决手写体数字识别,并比较几种算法(全连接、LeNet-5,LeNet-5+BN)在训练集上的精度变化,画出随epoch变化的曲线。
```python
import tensorflow as tf
from tensorflow.keras.datasets import mnist
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, BatchNormalization
# 加载MNIST数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train = x_train.reshape(x_train.shape[0], 28, 28, 1).astype('float32') / 255.
x_test = x_test.reshape(x_test.shape[0], 28, 28, 1).astype('float32') / 255.
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
# 构建全连接模型
fc_model = Sequential([
Flatten(input_shape=(28, 28, 1)),
Dense(units=128, activation='relu'),
Dense(units=64, activation='relu'),
Dense(units=10, activation='softmax')
])
# 构建LeNet-5模型
lenet5_model = Sequential([
Conv2D(filters=6, kernel_size=(5, 5), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D(pool_size=(2, 2)),
Conv2D(filters=16, kernel_size=(5, 5), activation='relu'),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(units=120, activation='relu'),
Dense(units=84, activation='relu'),
Dense(units=10, activation='softmax')
])
# 构建增加BN层的LeNet-5模型
lenet5_bn_model = Sequential([
Conv2D(filters=6, kernel_size=(5, 5), activation='relu', input_shape=(28, 28, 1)),
BatchNormalization(),
MaxPooling2D(pool_size=(2, 2)),
Conv2D(filters=16, kernel_size=(5, 5), activation='relu'),
BatchNormalization(),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(units=120, activation='relu'),
BatchNormalization(),
Dense(units=84, activation='relu'),
BatchNormalization(),
Dense(units=10, activation='softmax')
])
# 编译模型
fc_model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
lenet5_model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
lenet5_bn_model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
fc_history = fc_model.fit(x_train, y_train, batch_size=128, epochs=20, validation_data=(x_test, y_test))
lenet5_history = lenet5_model.fit(x_train, y_train, batch_size=128, epochs=20, validation_data=(x_test, y_test))
lenet5_bn_history = lenet5_bn_model.fit(x_train, y_train, batch_size=128, epochs=20, validation_data=(x_test, y_test))
# 画出随epoch变化的曲线
import matplotlib.pyplot as plt
plt.plot(fc_history.history['accuracy'])
plt.plot(lenet5_history.history['accuracy'])
plt.plot(lenet5_bn_history.history['accuracy'])
plt.title('Model accuracy')
plt.ylabel('Accuracy')
plt.xlabel('Epoch')
plt.legend(['FC', 'LeNet-5', 'LeNet-5+BN'], loc='lower right')
plt.show()
```
3. 分别搭建VGG13和ResNet18网络,解决CIFAR10数据集上的图片分类,画出这两种网络模型在该数据集上的随epoch的精度变化曲线。
```python
import tensorflow as tf
from tensorflow.keras.datasets import cifar10
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, BatchNormalization, Activation, Dropout
from tensorflow.keras.optimizers import SGD
from tensorflow.keras.callbacks import LearningRateScheduler
from tensorflow.keras import regularizers
import numpy as np
# 加载CIFAR10数据集
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
# 数据预处理
x_train = x_train.astype('float32') / 255.
x_test = x_test.astype('float32') / 255.
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
# 学习率衰减函数
def lr_schedule(epoch):
lr = 1e-3
if epoch > 75:
lr *= 0.5e-3
elif epoch > 50:
lr *= 1e-3
elif epoch > 25:
lr *= 1e-2
print('Learning rate:', lr)
return lr
# 构建VGG13模型
def vgg13_model():
model = Sequential([
Conv2D(64, (3, 3), padding='same', input_shape=x_train.shape[1:], activation='relu'),
BatchNormalization(),
Conv2D(64, (3, 3), padding='same', activation='relu'),
BatchNormalization(),
MaxPooling2D(pool_size=(2, 2)),
Dropout(0.2),
Conv2D(128, (3, 3), padding='same', activation='relu'),
BatchNormalization(),
Conv2D(128, (3, 3), padding='same', activation='relu'),
BatchNormalization(),
MaxPooling2D(pool_size=(2, 2)),
Dropout(0.3),
Conv2D(256, (3, 3), padding='same', activation='relu'),
BatchNormalization(),
Conv2D(256, (3, 3), padding='same', activation='relu'),
BatchNormalization(),
Conv2D(256, (3, 3), padding='same', activation='relu'),
BatchNormalization(),
MaxPooling2D(pool_size=(2, 2)),
Dropout(0.4),
Conv2D(512, (3, 3), padding='same', activation='relu'),
BatchNormalization(),
Conv2D(512, (3, 3), padding='same', activation='relu'),
BatchNormalization(),
Conv2D(512, (3, 3), padding='same', activation='relu'),
BatchNormalization(),
MaxPooling2D(pool_size=(2, 2)),
Dropout(0.5),
Flatten(),
Dense(512, activation='relu'),
BatchNormalization(),
Dropout(0.5),
Dense(10, activation='softmax')
])
return model
# 构建ResNet18模型
def resnet18_model():
def residual_block(inputs, filters, strides=1, projection=False):
identity = inputs
x = Conv2D(filters=filters, kernel_size=(3, 3), strides=strides, padding='same')(inputs)
x = BatchNormalization()(x)
x = Activation('relu')(x)
x = Conv2D(filters=filters, kernel_size=(3, 3), strides=1, padding='same')(x)
x = BatchNormalization()(x)
if projection:
identity = Conv2D(filters=filters, kernel_size=(1, 1), strides=strides, padding='same')(inputs)
identity = BatchNormalization()(identity)
x = tf.keras.layers.add([x, identity])
x = Activation('relu')(x)
return x
inputs = tf.keras.Input(shape=x_train.shape[1:])
x = Conv2D(filters=64, kernel_size=(7, 7), strides=2, padding='same')(inputs)
x = BatchNormalization()(x)
x = Activation('relu')(x)
x = MaxPooling2D(pool_size=(3, 3), strides=2, padding='same')(x)
x = residual_block(x, filters=64)
x = residual_block(x, filters=64)
x = residual_block(x, filters=128, strides=2, projection=True)
x = residual_block(x, filters=128)
x = residual_block(x, filters=256, strides=2, projection=True)
x = residual_block(x, filters=256)
x = residual_block(x, filters=512, strides=2, projection=True)
x = residual_block(x, filters=512)
x = tf.keras.layers.GlobalAveragePooling2D()(x)
x = Dense(units=10, activation='softmax')(x)
model = tf.keras.Model(inputs=inputs, outputs=x)
return model
# 编译模型
vgg13_model = vgg13_model()
resnet18_model = resnet18_model()
sgd = SGD(lr=lr_schedule(0), momentum=0.9)
vgg13_model.compile(optimizer=sgd, loss='categorical_crossentropy', metrics=['accuracy'])
resnet18_model.compile(optimizer=sgd, loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
vgg13_history = vgg13_model.fit(x_train, y_train, batch_size=128, epochs=30, validation_data=(x_test, y_test), callbacks=[LearningRateScheduler(lr_schedule)])
resnet18_history = resnet18_model.fit(x_train, y_train, batch_size=128, epochs=30, validation_data=(x_test, y_test), callbacks=[LearningRateScheduler(lr_schedule)])
# 画出随epoch变化的曲线
import matplotlib.pyplot as plt
plt.plot(vgg13_history.history['accuracy'])
plt.plot(resnet18_history.history['accuracy'])
plt.title('Model accuracy')
plt.ylabel('Accuracy')
plt.xlabel('Epoch')
plt.legend(['VGG13', 'ResNet18'], loc='lower right')
plt.show()
```
用jupyter写一个简单的图片识别界面,模型类型为pth
### 回答1:
首先,你需要安装PyTorch和Jupyter Notebook。安装方法可以参考PyTorch官方网站和Jupyter Notebook官方网站。
然后,你需要准备好要用来测试的图片和你训练的.pth模型。假设你已经将它们放在了同一个文件夹中。
接下来,打开Jupyter Notebook,在一个新的notebook中输入以下代码:
```python
import torch
import torchvision
import torchvision.transforms as transforms
from PIL import Image
import matplotlib.pyplot as plt
# 加载模型
model = torch.load('your_model.pth')
# 定义预处理函数
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
# 定义类别列表
classes = ['class1', 'class2', 'class3', ...]
# 定义预测函数
def predict(image_path):
# 加载图片
image = Image.open(image_path)
# 预处理图片
image = transform(image)
# 添加批次维度
image = image.unsqueeze(0)
# 将图片输入到模型中进行预测
output = model(image)
# 获取预测结果
_, predicted = torch.max(output.data, 1)
# 返回预测结果
return classes[predicted.item()]
# 加载图片并进行预测
image_path = 'your_image.jpg'
predicted_class = predict(image_path)
# 显示图片和预测结果
image = Image.open(image_path)
plt.imshow(image)
plt.title(predicted_class)
plt.show()
```
在代码中,我们首先加载了我们训练好的.pth模型,然后定义了一个预处理函数,用于将图片转换为模型输入需要的格式。接着,我们定义了一个预测函数,用于将图片输入到模型中进行预测,并返回预测结果。最后,我们加载了一张测试图片,并将其传入预测函数中进行预测,最终将预测结果显示出来。
注意,你需要将代码中的“your_model.pth”替换为你自己训练好的.pth模型的文件名,将“classes”列表替换为你自己的类别列表,将“your_image.jpg”替换为你自己的测试图片的文件名。
完成后,你可以运行代码并查看预测结果。
### 回答2:
要使用Jupyter写一个简单的图片识别界面,首先需要准备一个经过训练的.pth模型,并确保已安装好必要的Python库和Jupyter。
1. 打开Jupyter Notebook,并在一个新的笔记本中创建一个Cell。
2. 在该Cell中,首先导入所需的库:
```python
import torch
import torchvision.transforms as transforms
from PIL import Image
```
3. 将训练好的.pth模型加载到内存中:
```python
model = torch.load('your_model.pth')
```
4. 定义一个函数,用于预处理图像:
```python
def preprocess_image(image):
transform = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
image = transform(image).unsqueeze(0)
return image
```
5. 定义一个函数,用于进行图像识别:
```python
def predict_image(image_path):
image = Image.open(image_path)
image = preprocess_image(image)
output = model(image)
_, predicted = torch.max(output.data, 1)
return predicted.item()
```
6. 最后,定义界面的输入和输出部分:
```python
from IPython.display import display
from ipywidgets import FileUpload, Output, Image
upload = FileUpload()
output = Output()
display(upload)
def on_upload(change):
image_path = 'uploaded_image.jpg'
with open(image_path, 'wb') as file:
file.write(upload.data[0])
with output:
display(Image(value=upload.data[0], format='jpg', width=300, height=300))
prediction = predict_image(image_path)
print(f"图片识别结果为: {prediction}")
upload.observe(on_upload, names='_counter')
display(output)
```
7. 运行该Cell,就会在Jupyter界面中看到一个上传按钮。点击按钮,选择你要识别的图片进行上传,然后该图片将在界面上显示,并输出识别结果。
这样,你就用Jupyter写了一个简单的图片识别界面,可以使用.pth模型进行图像识别。
### 回答3:
Jupyter是一个开源的交互式编程环境,可以用于数据分析和可视化。如果我们想要在Jupyter中编写一个简单的图片识别界面,可以按照以下步骤进行操作:
1. 安装所需的库:首先,我们需要安装Pillow库来处理图像文件,以及Torchvision库来加载和处理模型;如果没有安装,可以使用以下命令安装:
```
!pip install Pillow
!pip install torchvision
```
2. 导入所需的库:在Jupyter笔记本的第一个单元格中,导入所需的库:
```python
import torch
import torch.nn as nn
import torchvision.transforms as transforms
import torchvision.models as models
from PIL import Image
```
3. 加载预训练模型:选择适合你的任务和数据集的模型,并加载预训练权重。例如,我们可以使用ResNet50模型:
```python
model = models.resnet50(pretrained=True)
```
4. 进行图像预处理:在对图像进行预测之前,我们需要对其进行正确的预处理。这包括将图像调整为模型的输入大小,将像素值标准化为0到1之间的范围,并将图像转换为Torch张量:
```python
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
def preprocess_image(image_path):
image = Image.open(image_path)
image = transform(image).unsqueeze(0)
return image
```
5. 进行预测:在Jupyter笔记本的一个单元格中,定义一个函数来进行图像预测,并根据模型的输出返回预测结果:
```python
def predict_image(image_path):
image = preprocess_image(image_path)
output = model(image)
_, predicted_idx = torch.max(output, 1)
return predicted_idx
```
6. 使用图像识别界面:在Jupyter笔记本中的另一个单元格中,创建一个用于上传图像文件的界面,并在上传成功后调用预测函数并显示结果:
```python
from IPython.display import display
import ipywidgets as widgets
uploader = widgets.FileUpload()
display(uploader)
def on_upload_button_clicked(b):
image_path = list(uploader.value.keys())[0]
predicted_idx = predict_image(image_path)
print(f"预测结果: {predicted_idx}")
upload_button = widgets.Button(description='上传图片')
upload_button.on_click(on_upload_button_clicked)
display(upload_button)
```
以上是在Jupyter中编写一个简单图像识别界面的基本步骤,你可以根据具体的需求和模型类型进行相应的修改和调整。
阅读全文
相关推荐
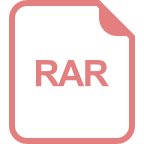
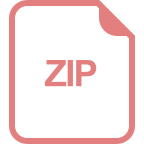
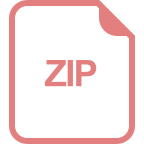
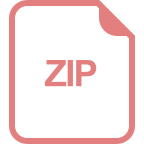
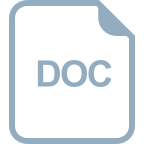
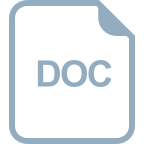
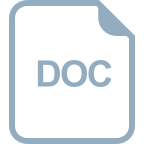
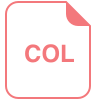
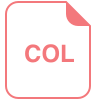
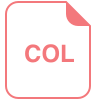
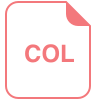
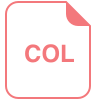
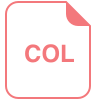
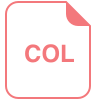


