编写一个循环队列类Java
时间: 2024-10-27 19:07:24 浏览: 26
编写一个循环队列(Circular Queue)在Java中,你可以创建一个类,它包含队列的底层数据结构(如数组),以及添加(enqueue)、删除(dequeue)、查看头部元素(front)和查看队列是否为空(isEmpty)等方法。这里是一个简单的实现:
```java
public class CircularQueue<T> {
private int capacity;
private T[] queue;
private int front;
private int rear;
// 初始化队列容量
public CircularQueue(int capacity) {
this.capacity = capacity;
this.queue = (T[]) new Object[capacity];
this.front = -1;
this.rear = -1;
}
// 添加元素到队尾
public boolean enqueue(T item) {
if ((rear + 1) % capacity == front) { // 队满
return false;
} else if (front == -1) { // 队空
front = rear = 0;
} else {
rear = (rear + 1) % capacity; // 循环更新 rear
}
queue[rear] = item;
return true;
}
// 从队头删除并返回元素
public T dequeue() {
if (isEmpty()) { // 队列空
throw new IllegalStateException("Queue is empty");
}
T removedItem = queue[front]; // 保存并移除首部元素
if (front == rear) { // 队列为单元素
front = rear = -1;
} else {
front = (front + 1) % capacity; // 更新 front
}
return removedItem;
}
// 查看队列是否为空
public boolean isEmpty() {
return front == -1 || front > rear;
}
// 返回队头元素,但不会删除
public T peek() {
if (isEmpty()) {
throw new IllegalStateException("Queue is empty");
}
return queue[front];
}
阅读全文
相关推荐
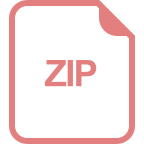
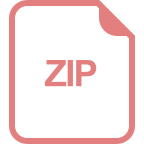
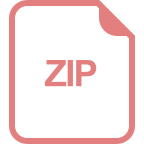
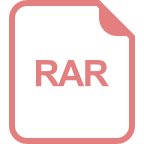
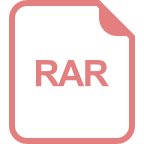
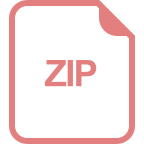
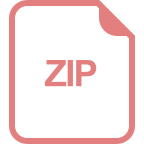
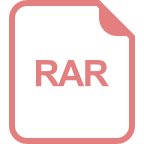
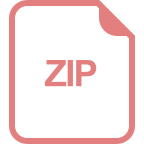
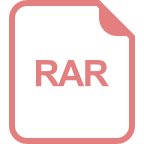
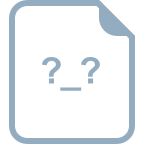
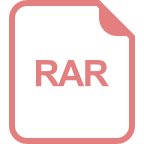
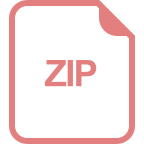
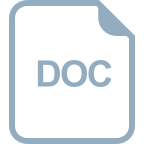
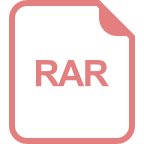
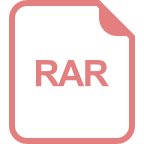
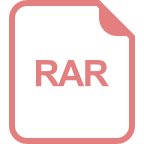
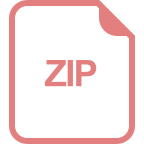
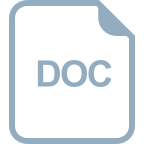