java实现移动Linux文件目录的文件
时间: 2024-09-19 18:09:24 浏览: 43
在Java中,你可以使用`java.io.File`类和`java.nio.file`包来操作Linux文件系统,包括移动或复制文件和目录。以下是一个基本的例子,展示了如何使用`Files.move()`或者`Files.copy()`方法来移动文件:
```java
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardCopyOption;
public class FileMoveExample {
public static void main(String[] args) {
// 源路径
String sourcePath = "/path/to/source/directory";
// 目标路径
String targetPath = "/path/to/destination/directory";
// 如果目标路径是个文件而不是目录,需要先创建它的父目录
Path targetFile = Paths.get(targetPath);
if (!Files.exists(targetFile.getParent())) {
Files.createDirectories(targetFile.getParent());
}
try {
// 移动文件
if (new File(sourcePath).isDirectory()) { // 判断是否为目录
// 使用Files.walk()遍历目录并移动每个文件
Files.walk(Paths.get(sourcePath), 1)
.filter(Files::isRegularFile) // 只处理普通文件
.forEach(file -> {
String fileName = file.getFileName().toString();
Path newPath = targetFile.resolve(fileName); // 新的完整路径
Files.move(file, newPath, StandardCopyOption.REPLACE_EXISTING);
});
} else {
// 移动单个文件
Path source = Paths.get(sourcePath);
Files.move(source, targetPath, StandardCopyOption.REPLACE_EXISTING);
}
} catch (Exception e) {
System.err.println("Error moving file or directory: " + e.getMessage());
}
}
}
```
阅读全文
相关推荐
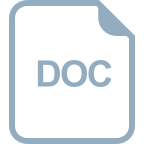
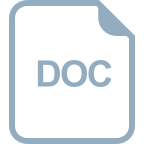
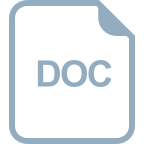
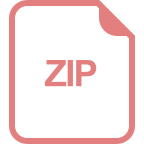
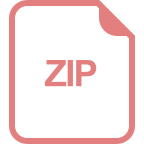
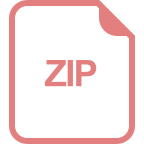
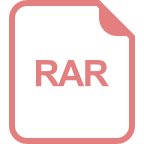
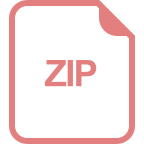
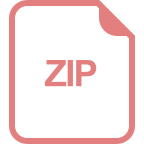
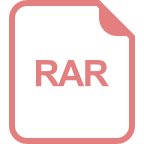
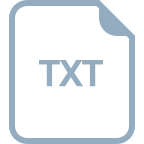
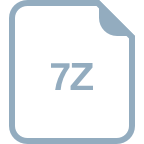
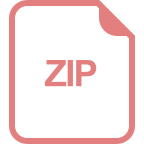
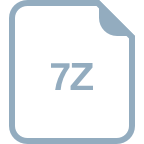
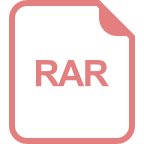
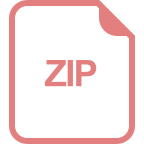
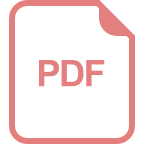
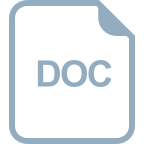
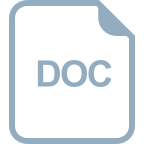