使用react实现一个语音转文字的效果
时间: 2024-10-27 08:08:09 浏览: 8
在React中实现语音转文字(也称为语音识别)通常需要结合Web Speech API,这是一个HTML5标准API,用于处理用户的音频输入。以下是步骤:
1. 首先,导入所需的库:如果使用的是React Native,可以考虑使用`react-native-web-speech-kit`等第三方库;如果是纯web应用,可以直接使用`window.speechRecognition`。
```jsx
import React, { useRef } from 'react';
```
2. 创建一个状态来存储识别结果和语音识别对象:
```jsx
const [transcription, setTranscription] = useState('');
const speechRecognition = useRef(new window.SpeechRecognition()).current;
```
3. 初始化语音识别对象并设置语言:
```jsx
useEffect(() => {
speechRecognition.lang = 'zh-CN'; // 设置为目标语言(例如中文)
}, []);
```
4. 开始录音和监听识别事件:
```jsx
const startRecording = async () => {
try {
await speechRecognition.start();
speechRecognition.onresult = (event) => {
const lastResult = event.results[event.results.length - 1];
if (lastResult.isFinal) {
setTranscription(lastResult[0].transcript);
}
};
} catch (error) {
console.error('Speech recognition error:', error);
}
};
```
5. 提供一个按钮或手势控制开始和停止录音:
```jsx
<button onClick={startRecording}>Start</button>
<button onClick={() => speechRecognition.stop()}>Stop</button>
```
6. 如果在React Native中使用,记得在`componentDidMount`、`componentWillUnmount`生命周期方法中初始化和清理语音识别资源。
这只是一个基础的示例,实际项目中可能还需要处理网络请求、错误处理以及用户交互的优化。同时,为了提高用户体验,可能还会添加加载指示器和错误提示。
阅读全文
相关推荐
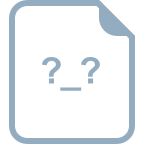
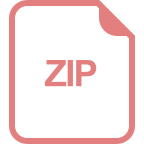
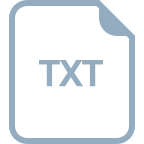
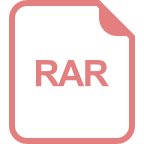
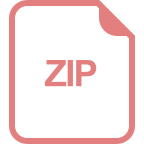
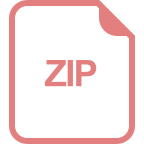
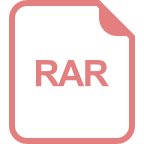
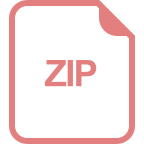
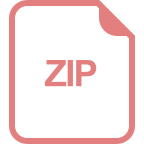
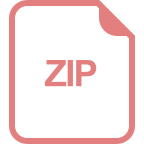
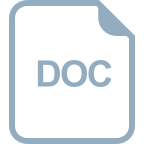
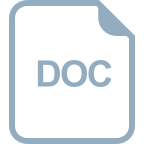
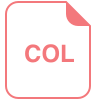
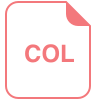
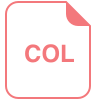
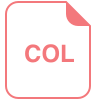
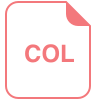
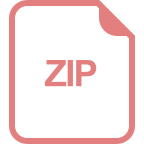
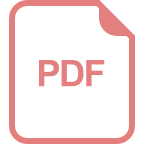