c#输入两个正整数:年份和月份,以空格分隔,输出这一年的这一月有多少天。需要考虑闰年。
时间: 2024-09-22 22:06:02 浏览: 75
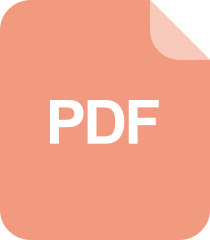
java 输入某年某月某日,判断这一天是这一年的第几天
在C#中,你可以使用DateTime类来处理日期相关的计算,包括判断是否为闰年以及计算某个月份的天数。下面是一个简单的函数示例,它接受用户输入的年份和月份,然后根据公历规则输出该月的天数:
```csharp
using System;
public class Program
{
public static void Main()
{
Console.Write("请输入年份(四位数)和月份(1-12):");
string input = Console.ReadLine();
int year, month;
// 尝试分割并转换输入值为整数
if (int.TryParse(input, out year) && year >= 0 && int.TryParse(input.Substring(input.IndexOf(' ') + 1), out month) && month >= 1 && month <= 12)
{
// 判断闰年
bool isLeapYear = IsLeapYear(year);
// 根据月份确定天数
int daysInMonth = GetDaysInMonth(month, isLeapYear);
Console.WriteLine($"{year}年的{month}月有{daysInMonth}天。");
}
else
{
Console.WriteLine("输入格式错误,请输入四个数字的年份和一个1到12的月份之间以空格分隔。");
}
}
// 判断是否为闰年
private static bool IsLeapYear(int year)
{
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 获取给定月份的天数
private static int GetDaysInMonth(int month, bool isLeapYear)
{
switch (month)
{
case 2:
return isLeapYear ? 29 : 28; // February has 29 days in a leap year and 28 otherwise.
case 4 case 6 case 9 case 11:
return 30; // April, June, September, and November have 30 days.
default:
return 31; // January, March, May, July, August, October, and December have 31 days.
}
}
}
```
阅读全文
相关推荐
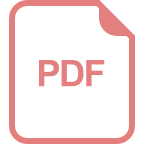
















