react中 div添加监听 resize 事件
时间: 2023-08-09 07:00:46 浏览: 86
在React中,我们可以使用class组件或函数组件将监听resize事件添加到div元素上。下面是使用class组件的示例:
1. 首先,在class组件的构造函数中初始化一个状态变量,用于保存div的宽度和高度:
```javascript
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
width: 0,
height: 0,
};
}
...
}
```
2. 接下来,在组件挂载完成后,我们可以通过ref获取到div元素的引用,并添加resize事件监听器:
```javascript
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
width: 0,
height: 0,
};
this.divRef = React.createRef();
}
componentDidMount() {
// 获取div元素引用
const div = this.divRef.current;
// 添加resize事件监听器
window.addEventListener('resize', this.handleResize);
// 更新div的初始宽度和高度
this.setState({
width: div.offsetWidth,
height: div.offsetHeight,
});
}
componentWillUnmount() {
// 移除resize事件监听器
window.removeEventListener('resize', this.handleResize);
}
handleResize = () => {
// 获取更新后的div宽度和高度
const div = this.divRef.current;
const width = div.offsetWidth;
const height = div.offsetHeight;
// 更新状态
this.setState({
width,
height,
});
}
render() {
return (
<div ref={this.divRef}>
{/* 其他组件内容 */}
</div>
);
}
}
```
通过以上步骤,我们成功地将resize事件监听添加到了div元素上,并且在窗口尺寸改变时更新了div的宽度和高度。
相关推荐
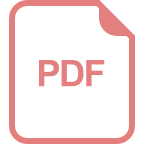
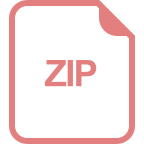
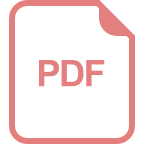














