<img class="image-size" :src="imgURL" alt="" @click="showBigImage = true" /> 图片是一个长方形的,怎么截取图片中间的正方形部分
时间: 2024-11-15 18:23:53 浏览: 4
要截取图片中间的正方形部分,你可以使用CSS来设置图片的显示方式。具体来说,可以通过设置`object-fit`属性为`cover`,并调整`object-position`属性来控制显示哪一部分的图片。
以下是一个简单的示例:
```html
<template>
<div class="image-container">
<img
class="image-size"
:src="imgURL"
alt=""
@click="showBigImage = true"
/>
</div>
</template>
<style scoped>
.image-container {
width: 300px; /* 容器宽度 */
height: 300px; /* 容器高度 */
overflow: hidden; /* 隐藏溢出的部分 */
}
.image-size {
width: 100%; /* 图片宽度 */
height: 100%; /* 图片高度 */
object-fit: cover; /* 保持图片比例,填充整个容器 */
object-position: center; /* 从图片中心开始显示 */
}
</style>
```
在这个示例中,`.image-container`是一个固定大小的容器,而`.image-size`是图片本身。通过设置`object-fit: cover`和`object-position: center`,可以确保图片以覆盖的方式填满容器,并且从图片的中心部分开始显示。这样,最终显示出来的就是一个位于图片中心的正方形部分。
如果你需要动态计算并截取图片的中间部分,可以使用JavaScript来实现。以下是一个更复杂的示例:
```html
<template>
<div class="image-container">
<img
class="image-size"
ref="image"
:src="imgURL"
alt=""
@click="showBigImage = true"
/>
</div>
</template>
<script>
export default {
data() {
return {
imgURL: 'your-image-url.jpg', // 替换为你的图片URL
showBigImage: false,
};
},
mounted() {
this.cropImage();
},
methods: {
cropImage() {
const img = this.$refs.image;
img.onload = () => {
const containerWidth = 300; // 容器宽度
const containerHeight = 300; // 容器高度
const aspectRatio = img.naturalWidth / img.naturalHeight;
let newWidth, newHeight;
if (aspectRatio > 1) {
newHeight = containerHeight;
newWidth = containerHeight * aspectRatio;
} else {
newWidth = containerWidth;
newHeight = containerWidth / aspectRatio;
}
const x = (newWidth - containerWidth) / 2;
const y = (newHeight - containerHeight) / 2;
img.style.width = `${newWidth}px`;
img.style.height = `${newHeight}px`;
img.style.objectFit = 'none';
img.style.objectPosition = `-${x}px -${y}px`;
};
},
},
};
</script>
<style scoped>
.image-container {
width: 300px; /* 容器宽度 */
height: 300px; /* 容器高度 */
overflow: hidden; /* 隐藏溢出的部分 */
}
.image-size {
display: block; /* 确保图片是块级元素 */
}
</style>
```
在这个示例中,我们使用JavaScript来计算图片的新尺寸,并通过设置`object-position`属性来截取图片的中间部分。这样可以确保无论图片的原始尺寸如何,最终显示的都是一个位于图片中心的正方形部分。
阅读全文
相关推荐
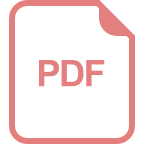
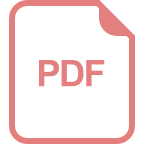
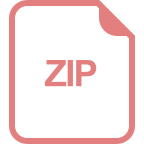
















