c++将字符串中的/替换为//
时间: 2023-10-04 22:07:41 浏览: 67
可以使用 C 语言中的字符串处理函数 `strchr()` 和 `strncpy()` 来实现将字符串中的 `/` 替换为 `//` 的操作。具体代码如下:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
int main() {
char str[MAX_LEN] = "hello/world/";
printf("Original string: %s\n", str);
char *p;
while ((p = strchr(str, '/')) != NULL) {
strncpy(p, "//", 2);
}
printf("Replaced string: %s\n", str);
return 0;
}
```
这段代码首先定义了一个字符串 `str`,然后使用 `strchr()` 函数在 `str` 中查找 `/` 字符,并将其替换为 `//`。循环直到所有的 `/` 字符都被替换完毕。最后输出替换后的字符串。
相关问题
c++ 替换字符串中的空格为_
你可以使用C++的string类和replace函数来替换字符串中的空格为下划线。下面是一个示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World! This is a test.";
std::string search = " ";
std::string replace = "_";
size_t pos = 0;
while ((pos = str.find(search, pos)) != std::string::npos) {
str.replace(pos, search.length(), replace);
pos += replace.length();
}
std::cout << str << std::endl;
return 0;
}
```
这段代码中,我们首先定义了一个字符串`str`,然后定义了要搜索的字符串`search`和要替换的字符串`replace`。接着使用`std::string`的`find`函数来查找字符串中的空格位置,并使用`replace`函数进行替换。通过循环,我们可以替换所有的空格。
运行这段代码后,输出将会是`Hello_World!_This_is_a_test.`,所有的空格都被替换为了下划线。
C++将wstring字符串\\转为/
可以使用以下代码将 `wstring` 字符串中的反斜杠转换为正斜杠:
```cpp
#include <algorithm>
#include <string>
std::wstring str = L"\\path\\to\\file";
std::replace(str.begin(), str.end(), L'\\', L'/');
```
其中,`std::replace` 函数用于将字符串中的某个字符替换为另一个字符。在这个例子中,将 `\\` 替换为 `/`。
相关推荐
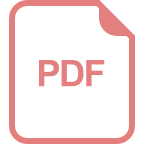
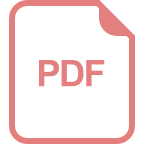
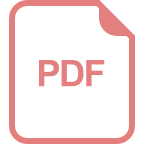












